Video manipulation and delivery
Cloudinary is a cloud-based service that provides an end-to-end media management solution for images and videos, including upload, storage, administration, manipulation, optimization and delivery.
Using Cloudinary's comprehensive API and easy to use manipulation URLs, uploaded videos can be automatically converted to all relevant formats suitable for web viewing, optimized for web browsers and mobile devices, normalized and manipulated in real time to fit your graphic design. The videos can be easily embedded in your website using HTML5 video tags (or Flash) and then streamed to your users via a fast CDN (see the Upload videos documentation for more information on uploading videos).
Cloudinary’s video management service enables simple video delivery and advanced video manipulation as follows:
- Stream videos using simple URLs
- Embed videos in web pages
- Resize the video dimensions and crop to match your graphic design
- Trim the video and specify the section to keep
- Concatenate videos together
- Rotate the video
- Round corners and create circular videos
- Adjust the video settings: format, quality, bit rate and codec
- Apply a filter or control video effects such as brightness, contrast, etc
- Control the audio codec and frequency, or remove the audio channel
- Generate image thumbnails of any frame of the video, and further manipulate the image
- Generate waveform images from an audio file or the audio track of a video
- Add watermarks and image overlays
- Add video overlays
- Create dynamic text captions
- Add subtitles
- Apply a 3D LUT file to your video
- Enable Adaptive bitrate streaming - HLS and MPEG-DASH
- Manipulate and stream audio
- Convert the video to an animated GIF or animated WebP
The Reference table at the bottom of this page summarizes all parameters available for manipulating videos.
Streaming videos using URLs
Accessing uploaded videos is done using simple Cloudinary delivery HTTP or HTTPS URLs which are then streamed to users via a worldwide fast CDN. The URL contains the public ID of the requested video plus any optional manipulation parameters. Public ID is the unique identifier of the video, and is either specified when uploading the video to your Cloudinary account, or automatically assigned by Cloudinary (see Remote Upload for more details on the various options for specifying the public ID).
The video delivery URL takes the following structure:
http://res.cloudinary.com/<cloud name>/video/upload/<public ID>.<video format file extension>
Note: For customers with a Custom Domain Name (CNAME - available for Cloudinary's Advanced plan and above), the video delivery URL becomes:
http://<custom domain name>/video/upload/<public ID>.<video format file extension>
For example, displaying the mp4 video with a public ID of dog
uploaded to Cloudinary’s demo account:
cl_video_tag("dog")
cl_video_tag("dog")
CloudinaryVideo("dog").video()
cloudinary.video("dog")
cloudinary.url().videoTag("dog")
$.cloudinary.video("dog")
cloudinary.Api.UrlVideoUp.BuildVideoTag("dog")
Any manipulation (transformation) instructions can be easily added before the public ID in the delivery URL (see the Video transformations reference table for more details on possible values). The video delivery URL with manipulation parameters takes the following structure:
http://res.cloudinary.com/<cloud name>/video/upload/<manipulation parameters>/<public ID>.<video format file extension>
An example of a URL with video manipulation, where the mp4 video with a public ID of dog
is cropped to a width of 300 pixels and a height of 200 pixels:
cl_video_tag("dog", :width=>300, :height=>200, :crop=>"crop")
cl_video_tag("dog", array("width"=>300, "height"=>200, "crop"=>"crop"))
CloudinaryVideo("dog").video(width=300, height=200, crop="crop")
cloudinary.video("dog", {width: 300, height: 200, crop: "crop"})
cloudinary.url().transformation(new Transformation().width(300).height(200).crop("crop")).videoTag("dog")
$.cloudinary.video("dog", {width: 300, height: 200, crop: "crop"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(300).Height(200).Crop("crop")).BuildVideoTag("dog")
Note: you can deliver the video as webm, mp4, ogv or flv by simply changing the video format file extension (see Transcoding videos for more details).
Embedding videos in web pages
You can use Cloudinary’s web framework SDKs to add videos to your web application and simplify creating manipulation URLs and embedding HTML5 video tags with multiple video sources and settings.
The Cloudinary video tag helper method (e.g. cl_video_tag
in Ruby on Rails) automatically generates an HTML5 video tag including the video source URLs of all relevant formats supported by web browsers (webm
, mp4
and ogv
), as well as a poster thumbnail image. The browser then automatically selects the video format it supports, while the video files are created dynamically when first accessed by your users.
You can optionally control the generation of the HTML5 video tag and specify transformation parameters to manipulate the video to match your graphic design. The video tag helper method uses the following structure:
cl_video_tag(public_id, options = {})
public_id
: (required) the unique identifier of the uploaded video.
Where possible options are:
source_types
: an ordered array of the video source types to include in the HTML5 tag, where the type is mapped to the mime type. Default:['webm', 'mp4', 'ogv']
manipulation params
: the manipulation(s) to carry out on the video, such as cropping, resizing, etc (see the Video transformations reference table for more details on possible parameters and values)source_transformation
: to override the default transformation instructions for each specific video format. Thesource_type
is mapped to a transformation struct. e.g.,{ mp4: { quality: 70}}
poster
: a URI to an image to be shown while the video is downloading or until the user hits the play button. The URI can be one of the following:- The URL to an image.
- A struct including the transformations to apply to the default image (you can include the public_id of an uploaded image to use instead of the default image).
- Set to
nil
orfalse
to use no poster. - Default: the middle frame of the source video in jpg format.
fallback_content
: an HTML string to display in the case that the browser does not support any of the video formats included.- Any attribute of the HTML5 video tag. e.g.,
autoplay
,controls
,height
,loop
,muted
,preload
(see w3schools for details on HTML5 attributes).
Example 1: Simply creating an HTML5 video tag for the uploaded mp4 video named dog
:
cl_video_tag("dog")
<?php echo cl_video_tag("dog"); ?>
cloudinary.CloudinaryVideo("dog").video()
cloudinary.video("dog")
<cl:video src="dog"/>
Generates the following HTML5 video tag:
<video poster="http://res.cloudinary.com/demo/video/upload/dog.jpg"> <source src="http://res.cloudinary.com/demo/image/upload/dog.webm" type="video/webm"/> <source src="http://res.cloudinary.com/demo/image/upload/dog.mp4" type="video/mp4"/> <source src="http://res.cloudinary.com/demo/image/upload/dog.ogv" type="video/ogg"/> </video>
Example 2: Creating an HTML5 video tag for the uploaded mp4 video named dog
, where the video is resized to a width of 300 pixels, a height of 300 pixels, padded with a blue background to fill the new dimensions, given a fallback string in the case that the video tag is not supported, specified that controls should be displayed and that the file should not be preloaded:
cl_video_tag("dog", :width => 300, :height => 300, :crop => :pad, :background => "blue", :preload => "none", :controls => true, :fallback_content => "Your browser does not support HTML5 video tags" )
<?php echo cl_video_tag("dog", array("width" => 300, "height" => 300, "crop" => "pad", "background" => "blue", "preload" => "none", "controls" => true, "fallback_content" => "Your browser does not support HTML5 video tags")); ?>
cloudinary.CloudinaryVideo("dog").video( width = 300, height = 300, crop = "pad", background = "blue", preload = "none", controls = True, fallback_content = "Your browser does not support HTML5 video tags")
cloudinary.video("dog" { width: 300, height: 300, crop: "pad", background: "blue", preload: "none", controls: true, fallback_content: "Your browser does not support HTML5 video tags" })
<cl:video src="dog" width = "300", height = "300", crop = "pad", background = "blue", preload = "none", controls = "true", fallback_content = "Your browser does not support HTML5 video tags"/>
Generates the following HTML5 video tag:
<video poster="http://res.cloudinary.com/demo/video/upload/dog.jpg" preload="none" controls> <source src="http://res.cloudinary.com/demo/video/upload/w_300,h_300,c_pad,b_blue/dog.webm" type="video/webm"/> <source src="http://res.cloudinary.com/demo/video/upload/w_300,h_300,c_pad,b_blue/dog.mp4" type="video/mp4"/> <source src="http://res.cloudinary.com/demo/video/upload/w_300,h_300,c_pad,b_blue/dog.ogv" type="video/ogg"/> Your browser does not support HTML5 video tags </video>
Example 3: Creating an HTML5 video tag for the uploaded mp4 video named dog
, where the video is cropped to a width of 200 pixels and a height of 150 pixels, given a fallback string in the case that the video tag is not supported, not to use a poster image and that the file should autoplay in a loop:
cl_video_tag("dog", :width => 200, :height => 150, :crop => :crop, :loop => true, :autoplay => true, :poster => false, :fallback_content => "Your browser does not support HTML5 video tags" )
<?php echo cl_video_tag("dog", array("width" => 200, "height" => 150, "crop" => "crop", "loop" => true, "autoplay" => true, "poster" => false, "fallback_content" => "Your browser does not support HTML5 video tags")); ?>
cloudinary.CloudinaryVideo("dog").video( width = 200, height = 150, crop = "crop", loop = True, autoplay = True, poster = False, fallback_content = "Your browser does not support HTML5 video tags")
cloudinary.video("dog" { width: 200, height: 150, crop: "crop", loop: true, autoplay: true, poster: false, fallback_content: "Your browser does not support HTML5 video tags" })
<cl:video src="dog" width = "200", height = "150", crop = "crop", loop = "true", autoplay = "true", poster = "false", fallback_content = "Your browser does not support HTML5 video tags"/>
Generates the following HTML5 video tag:
<video loop autoplay> <source src="http://res.cloudinary.com/demo/video/upload/w_200,h_150,c_crop/dog.webm" type="video/webm"/> <source src="http://res.cloudinary.com/demo/video/upload/w_200,h_150,c_crop/dog.mp4" type="video/mp4"/> <source src="http://res.cloudinary.com/demo/video/upload/w_200,h_150,c_crop/dog.ogv" type="video/ogg"/> Your browser does not support HTML5 video tags </video>
Resizing and cropping videos
You can resize and crop videos in order to match the graphic design of your web site or mobile app. Whether videos are uploaded in your server-side code or by your users, the originals are stored in the cloud. You can then dynamically create multiple resized, cropped and manipulated videos on-the-fly and deliver them via dynamic URLs or use code to either add video tags or build the URLs.
Change the size of a video with the width
and height
parameters (w
and h
in URLs). You can resize the video by using both the width and height parameters or with only one of them (the other dimension is automatically updated to maintain the aspect ratio).
- Using an integer value sets the new dimension to that number in pixels. For example,
w_150
sets the width to exactly 150 pixels. - Using a decimal value sets the new dimension as a multiple of the original dimension. For example,
w_0.5
sets the width to half the original width.
Examples of resizing the uploaded mp4 video named dog
:
Resizing to a width of 150 pixels and a height of 100 pixels:
Ruby:cl_video_tag("dog", :width=>150, :height=>100)
PHP:cl_video_tag("dog", array("width"=>150, "height"=>100))
Python:CloudinaryVideo("dog").video(width=150, height=100)
Node.js:cloudinary.video("dog", {width: 150, height: 100})
Java:cloudinary.url().transformation(new Transformation().width(150).height(100)).videoTag("dog")
jQuery:$.cloudinary.video("dog", {width: 150, height: 100})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(150).Height(100)).BuildVideoTag("dog")
Resizing the width to half the original width, maintaining the aspect ratio:
Ruby:cl_video_tag("dog", :width=>0.5)
PHP:cl_video_tag("dog", array("width"=>0.5))
Python:CloudinaryVideo("dog").video(width=0.5)
Node.js:cloudinary.video("dog", {width: 0.5})
Java:cloudinary.url().transformation(new Transformation().width(0.5)).videoTag("dog")
jQuery:$.cloudinary.video("dog", {width: 0.5})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(0.5)).BuildVideoTag("dog")
Resizing the height to 200 pixels, maintaining the aspect ratio:
Ruby:cl_video_tag("dog", :height=>200)
PHP:cl_video_tag("dog", array("height"=>200))
Python:CloudinaryVideo("dog").video(height=200)
Node.js:cloudinary.video("dog", {height: 200})
Java:cloudinary.url().transformation(new Transformation().height(200)).videoTag("dog")
jQuery:$.cloudinary.video("dog", {height: 200})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().Height(200)).BuildVideoTag("dog")
When changing the dimensions of an uploaded video by manipulating the video's height and/or width, you need to decide how to adapt or "crop" the video to fit into the requested size. Use the crop
parameter for selecting the crop mode (c
in URLs). Cloudinary supports the following video cropping modes: scale, fit, fill, limit, pad, lpad and crop.
scale
Change the size of the video exactly to the given width and height without necessarily retaining the original aspect ratio: all original video parts are visible but might be stretched or shrunk. If only the width or height is given, then the video is scaled to the new dimension while retaining the original aspect ratio (the other dimension is also scaled proportionately). This is the default cropping mode for resizing videos if the crop mode is not specified.
For example, the uploaded mp4 video named dog
scaled to a width of 150 pixels while maintaining aspect ratio:
cl_video_tag("dog", :width=>150, :crop=>"scale")
cl_video_tag("dog", array("width"=>150, "crop"=>"scale"))
CloudinaryVideo("dog").video(width=150, crop="scale")
cloudinary.video("dog", {width: 150, crop: "scale"})
cloudinary.url().transformation(new Transformation().width(150).crop("scale")).videoTag("dog")
$.cloudinary.video("dog", {width: 150, crop: "scale"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(150).Crop("scale")).BuildVideoTag("dog")
fit
Change video size to fit in the given width and height while retaining the original aspect ratio with all the original video parts visible. The new dimensions of the transformed video must not exceed the specified width and/or height, but may be enlarged if the video is smaller than the new dimensions. However, the video may be smaller than the specified values in order to keep the original proportions.
For example, the uploaded mp4 video named dog
fitted to a width and height of 250 pixels while retaining the aspect ratio:
cl_video_tag("dog", :width=>250, :height=>250, :crop=>"fit")
cl_video_tag("dog", array("width"=>250, "height"=>250, "crop"=>"fit"))
CloudinaryVideo("dog").video(width=250, height=250, crop="fit")
cloudinary.video("dog", {width: 250, height: 250, crop: "fit"})
cloudinary.url().transformation(new Transformation().width(250).height(250).crop("fit")).videoTag("dog")
$.cloudinary.video("dog", {width: 250, height: 250, crop: "fit"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(250).Height(250).Crop("fit")).BuildVideoTag("dog")
fill
Create a video with the exact given width and height while retaining the original aspect ratio, using only part of the video that fills the given dimensions if necessary (only part of the original video might be visible if the requested aspect ratio is different to the original aspect ratio). You can also specify which part of the original video to use for filling the required dimensions in case the proportions do not match by using the gravity parameter.
For example, the uploaded mp4 video named dog
filled to a width and height of 250 pixels while retaining the aspect ratio:
cl_video_tag("dog", :width=>250, :height=>250, :crop=>"fill")
cl_video_tag("dog", array("width"=>250, "height"=>250, "crop"=>"fill"))
CloudinaryVideo("dog").video(width=250, height=250, crop="fill")
cloudinary.video("dog", {width: 250, height: 250, crop: "fill"})
cloudinary.url().transformation(new Transformation().width(250).height(250).crop("fill")).videoTag("dog")
$.cloudinary.video("dog", {width: 250, height: 250, crop: "fill"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(250).Height(250).Crop("fill")).BuildVideoTag("dog")
limit
The limit mode is used for creating a video that does not exceed the given width and height. If the original video is smaller than the given limits, the generated video uses the same dimensions as the original one. If the original is bigger than the given limits, it will be scaled down to fit the given dimensions while retaining original proportions (similar to the fit
mode in this case).
For example, the uploaded mp4 video named dog
limited to a width and height of 250 pixels while retaining the aspect ratio:
cl_video_tag("dog", :width=>250, :height=>250, :crop=>"limit")
cl_video_tag("dog", array("width"=>250, "height"=>250, "crop"=>"limit"))
CloudinaryVideo("dog").video(width=250, height=250, crop="limit")
cloudinary.video("dog", {width: 250, height: 250, crop: "limit"})
cloudinary.url().transformation(new Transformation().width(250).height(250).crop("limit")).videoTag("dog")
$.cloudinary.video("dog", {width: 250, height: 250, crop: "limit"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(250).Height(250).Crop("limit")).BuildVideoTag("dog")
pad
Resize the video to fill the given width and height while retaining the original aspect ratio.
If the proportions of the original video do not match the required ones, padding is added to the video to reach the required size. You can also specify which part of the original video to use for filling the required dimensions in case the proportions do not match by using the gravity parameter, and specify the color of the background in the case that padding is added.
For example, the uploaded mp4 video named dog
padded to a width and height of 250 pixels while retaining the aspect ratio:
cl_video_tag("dog", :width=>250, :height=>250, :background=>"black", :crop=>"pad")
cl_video_tag("dog", array("width"=>250, "height"=>250, "background"=>"black", "crop"=>"pad"))
CloudinaryVideo("dog").video(width=250, height=250, background="black", crop="pad")
cloudinary.video("dog", {width: 250, height: 250, background: "black", crop: "pad"})
cloudinary.url().transformation(new Transformation().width(250).height(250).background("black").crop("pad")).videoTag("dog")
$.cloudinary.video("dog", {width: 250, height: 250, background: "black", crop: "pad"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(250).Height(250).Background("black").Crop("pad")).BuildVideoTag("dog")
lpad
Same as the pad
mode above, but doesn't scale the video up if your requested dimensions are larger than the original video's.
For example, the uploaded mp4 video named dog
lpadded to a width and height of 250 pixels while retaining the aspect ratio and limiting the size to no larger than the original video:
cl_video_tag("dog", :width=>250, :height=>250, :background=>"black", :crop=>"lpad")
cl_video_tag("dog", array("width"=>250, "height"=>250, "background"=>"black", "crop"=>"lpad"))
CloudinaryVideo("dog").video(width=250, height=250, background="black", crop="lpad")
cloudinary.video("dog", {width: 250, height: 250, background: "black", crop: "lpad"})
cloudinary.url().transformation(new Transformation().width(250).height(250).background("black").crop("lpad")).videoTag("dog")
$.cloudinary.video("dog", {width: 250, height: 250, background: "black", crop: "lpad"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(250).Height(250).Background("black").Crop("lpad")).BuildVideoTag("dog")
crop
Extract only part of a given width and height out of the original video. The original proportions are retained and you can also specify which part of the original video to use for filling the required dimensions by giving the x
and y
coordinates of the top left corner, or by using the gravity parameter.
For example, cropping the uploaded mp4 video named dog
to a width of 350 pixels and a height of 200 pixels, starting at the x = 230 pixels and y = 20 pixels coordinate:
cl_video_tag("dog", :width=>350, :height=>200, :x=>230, :y=>20, :crop=>"crop")
cl_video_tag("dog", array("width"=>350, "height"=>200, "x"=>230, "y"=>20, "crop"=>"crop"))
CloudinaryVideo("dog").video(width=350, height=200, x=230, y=20, crop="crop")
cloudinary.video("dog", {width: 350, height: 200, x: 230, y: 20, crop: "crop"})
cloudinary.url().transformation(new Transformation().width(350).height(200).x(230).y(20).crop("crop")).videoTag("dog")
$.cloudinary.video("dog", {width: 350, height: 200, x: 230, y: 20, crop: "crop"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(350).Height(200).X(230).Y(20).Crop("crop")).BuildVideoTag("dog")
Aspect ratio
Use the aspect_ratio
parameter (ar
in URLs) to resize the video to a new aspect ratio. This parameter is used together with a specified crop mode that determines how the video is adjusted to the new dimensions. This parameter can also be used when changing the dimensions of a video with only the width
or height
parameters (w
and h
in URLs) - the other dimension is then automatically updated to maintain the given aspect ratio.
The aspect_ratio
parameter accepts a value in one of the following forms:
a:b
wherea
signifies the relative width andb
the relative height (e.g., 4:3 or 16:9).- a decimal value representing the ratio of the width divided by the height (e.g., 1.33 or 2.5).
Examples with the uploaded mp4 video named dog
:
Cropped to an aspect ratio of 2.5:
Ruby:cl_video_tag("dog", :aspect_ratio=>"2.5", :crop=>"crop")
PHP:cl_video_tag("dog", array("aspect_ratio"=>"2.5", "crop"=>"crop"))
Python:CloudinaryVideo("dog").video(aspect_ratio="2.5", crop="crop")
Node.js:cloudinary.video("dog", {aspect_ratio: "2.5", crop: "crop"})
Java:cloudinary.url().transformation(new Transformation().aspectRatio("2.5").crop("crop")).videoTag("dog")
jQuery:$.cloudinary.video("dog", {aspect_ratio: "2.5", crop: "crop"})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().AspectRatio("2.5").Crop("crop")).BuildVideoTag("dog")
Filled to a width of 400 pixels with an aspect ratio of 4:3:
Ruby:cl_video_tag("dog", :width=>400, :aspect_ratio=>"4:3", :crop=>"fill")
PHP:cl_video_tag("dog", array("width"=>400, "aspect_ratio"=>"4:3", "crop"=>"fill"))
Python:CloudinaryVideo("dog").video(width=400, aspect_ratio="4:3", crop="fill")
Node.js:cloudinary.video("dog", {width: 400, aspect_ratio: "4:3", crop: "fill"})
Java:cloudinary.url().transformation(new Transformation().width(400).aspectRatio("4:3").crop("fill")).videoTag("dog")
jQuery:$.cloudinary.video("dog", {width: 400, aspect_ratio: "4:3", crop: "fill"})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(400).AspectRatio("4:3").Crop("fill")).BuildVideoTag("dog")
Gravity
Gravity specifies which part of the original video to use for certain cropping modes, for filling the required dimensions in case the proportions do not match. This is accomplished by using the gravity
parameter (g
in URLs). The supported gravity values are: north_east
, north
, north_west
, west
, south_west
, south
, south_east
, east
, or center
(the default value).
For example, the uploaded mp4 video named dog
filled to a width and height of 250 pixels while retaining the aspect ratio, with gravity set to north:
cl_video_tag("dog", :width=>250, :height=>250, :gravity=>"north", :crop=>"fill")
cl_video_tag("dog", array("width"=>250, "height"=>250, "gravity"=>"north", "crop"=>"fill"))
CloudinaryVideo("dog").video(width=250, height=250, gravity="north", crop="fill")
cloudinary.video("dog", {width: 250, height: 250, gravity: "north", crop: "fill"})
cloudinary.url().transformation(new Transformation().width(250).height(250).gravity("north").crop("fill")).videoTag("dog")
$.cloudinary.video("dog", {width: 250, height: 250, gravity: "north", crop: "fill"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(250).Height(250).Gravity("north").Crop("fill")).BuildVideoTag("dog")
Background color
Use the background
parameter (b
in URLs) to set the color in the case of padding added with the “pad” crop mode. The color can be set as an RGB hex triplet (e.g. b_rgb:3e2222
), a 3 character RGB hex (e.g. b_rgb:777
) or a named color (e.g. b_green
). Cloudinary's client libraries also support a #
shortcut for RGB (e.g. setting background
to #3e2222
which is then translated to b_rgb:3e2222
).
For example, the uploaded mp4 video named dog
padded to a width and height of 300 pixels with a green background:
cl_video_tag("dog", :width=>300, :height=>300, :background=>"green", :crop=>"pad")
cl_video_tag("dog", array("width"=>300, "height"=>300, "background"=>"green", "crop"=>"pad"))
CloudinaryVideo("dog").video(width=300, height=300, background="green", crop="pad")
cloudinary.video("dog", {width: 300, height: 300, background: "green", crop: "pad"})
cloudinary.url().transformation(new Transformation().width(300).height(300).background("green").crop("pad")).videoTag("dog")
$.cloudinary.video("dog", {width: 300, height: 300, background: "green", crop: "pad"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(300).Height(300).Background("green").Crop("pad")).BuildVideoTag("dog")
Trimming videos
Trim a video (and discard the rest) by using a combination of the following 3 parameters to specify the section of video to keep after trimming:
start_offset
(so
in URLs) specifies the start.end_offset
(eo
in URLs) specifies the end.duration
(du
in URLs) specifies the duration.
Possible values:
- A float representing the time in seconds e.g.
5.44
(5 seconds and 440 milliseconds) - A string representing the percentage of the video length. This string consists of a number with a
p
appended e.g.35p
(0p is the first frame and 100p is the last frame). The client libraries also support appending a%
instead of ap
.
Examples with the uploaded mp4 video named dog
:
Trimming the video to the section that starts at 6.5 seconds and ends at 10 seconds:
Ruby:cl_video_tag("dog", :start_offset=>"6.5", :end_offset=>"10")
PHP:cl_video_tag("dog", array("start_offset"=>"6.5", "end_offset"=>"10"))
Python:CloudinaryVideo("dog").video(start_offset="6.5", end_offset="10")
Node.js:cloudinary.video("dog", {start_offset: "6.5", end_offset: "10"})
Java:cloudinary.url().transformation(new Transformation().startOffset("6.5").endOffset("10")).videoTag("dog")
jQuery:$.cloudinary.video("dog", {start_offset: "6.5", end_offset: "10"})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().StartOffset("6.5").EndOffset("10")).BuildVideoTag("dog")
Trimming the video to the section that starts at 10 percent with a duration of 30 percent (effectively from the 10 percent mark to the 40 percent mark):
Ruby:cl_video_tag("dog", :start_offset=>"10p", :duration=>"30p")
PHP:cl_video_tag("dog", array("start_offset"=>"10p", "duration"=>"30p"))
Python:CloudinaryVideo("dog").video(start_offset="10p", duration="30p")
Node.js:cloudinary.video("dog", {start_offset: "10p", duration: "30p"})
Java:cloudinary.url().transformation(new Transformation().startOffset("10p").duration("30p")).videoTag("dog")
jQuery:$.cloudinary.video("dog", {start_offset: "10p", duration: "30p"})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().StartOffset("10p").Duration("30p")).BuildVideoTag("dog")
Client libraries also support the offset
shortcut parameter to combine the 2 values representing the start and end of the video to keep e.g. [2.5, 9.5]
or ['10p', '30p']
. In frameworks that support ranges you can use: 2.66..3.21
or '35%..70%'
. An equivalent of the offset shortcut is not available for URLs.
For example, trimming the uploaded mp4 video named dog
to the section that starts at 6.5 seconds and ends at 10 seconds:
cl_video_tag("dog", :start_offset=>"6.5", :end_offset=>"10")
cl_video_tag("dog", array("start_offset"=>"6.5", "end_offset"=>"10"))
CloudinaryVideo("dog").video(start_offset="6.5", end_offset="10")
cloudinary.video("dog", {start_offset: "6.5", end_offset: "10"})
cloudinary.url().transformation(new Transformation().startOffset("6.5").endOffset("10")).videoTag("dog")
$.cloudinary.video("dog", {start_offset: "6.5", end_offset: "10"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().StartOffset("6.5").EndOffset("10")).BuildVideoTag("dog")
Concatenating videos
Cloudinary supports the concatenation of videos by using the overlay video parameter (l_video:
in URLs) to specify the name of another uploaded video and then the splice
flag (fl_splice
in URLs) to indicate that the video should be concatenated on to the container video and not added as an overlay.
Note that the videos spliced together must be the same width and height. You can use size transformation parameters to ensure that both videos match in size (w
and h
).
For example, to concatenate the video named dog
on to the end of the video named kitten_fighting
, with both videos set to a width of 300 pixels and a height of 200 pixels:
cl_video_tag("kitten_fighting", :transformation=>[ {:width=>300, :height=>200, :crop=>"fill"}, {:overlay=>"video:dog", :flags=>"splice", :width=>300, :height=>200, :crop=>"fill"} ])
cl_video_tag("kitten_fighting", array("transformation"=>array( array("width"=>300, "height"=>200, "crop"=>"fill"), array("overlay"=>"video:dog", "flags"=>"splice", "width"=>300, "height"=>200, "crop"=>"fill") )))
CloudinaryVideo("kitten_fighting").video(transformation=[ {"width": 300, "height": 200, "crop": "fill"}, {"overlay": "video:dog", "flags": "splice", "width": 300, "height": 200, "crop": "fill"} ])
cloudinary.video("kitten_fighting", {transformation: [ {width: 300, height: 200, crop: "fill"}, {overlay: "video:dog", flags: "splice", width: 300, height: 200, crop: "fill"} ]})
cloudinary.url().transformation(new Transformation() .width(300).height(200).crop("fill").chain() .overlay("video:dog").flags("splice").width(300).height(200).crop("fill")).videoTag("kitten_fighting")
$.cloudinary.video("kitten_fighting", {transformation: [ {width: 300, height: 200, crop: "fill"}, {overlay: "video:dog", flags: "splice", width: 300, height: 200, crop: "fill"} ]})
cloudinary.Api.UrlVideoUp.Transform(new Transformation() .Width(300).Height(200).Crop("fill").Chain() .Overlay("video:dog").Flags("splice").Width(300).Height(200).Crop("fill")).BuildVideoTag("kitten_fighting")
By default, the video is spliced on to the end of the container video: to splice the video on to the beginning, add the start_offset
parameter and set it to 0 (so_0
in URLs). For example, to concatenate the video named dog
on to the beginning of the video named kitten_fighting
, with both videos set to a width of 300 pixels and a height of 200 pixels:
cl_video_tag("kitten_fighting", :transformation=>[ {:width=>300, :height=>200, :crop=>"fill"}, {:overlay=>"video:dog", :flags=>"splice", :width=>300, :height=>200, :start_offset=>"0", :crop=>"fill"} ])
cl_video_tag("kitten_fighting", array("transformation"=>array( array("width"=>300, "height"=>200, "crop"=>"fill"), array("overlay"=>"video:dog", "flags"=>"splice", "width"=>300, "height"=>200, "start_offset"=>"0", "crop"=>"fill") )))
CloudinaryVideo("kitten_fighting").video(transformation=[ {"width": 300, "height": 200, "crop": "fill"}, {"overlay": "video:dog", "flags": "splice", "width": 300, "height": 200, "start_offset": "0", "crop": "fill"} ])
cloudinary.video("kitten_fighting", {transformation: [ {width: 300, height: 200, crop: "fill"}, {overlay: "video:dog", flags: "splice", width: 300, height: 200, start_offset: "0", crop: "fill"} ]})
cloudinary.url().transformation(new Transformation() .width(300).height(200).crop("fill").chain() .overlay("video:dog").flags("splice").width(300).height(200).startOffset("0").crop("fill")).videoTag("kitten_fighting")
$.cloudinary.video("kitten_fighting", {transformation: [ {width: 300, height: 200, crop: "fill"}, {overlay: "video:dog", flags: "splice", width: 300, height: 200, start_offset: "0", crop: "fill"} ]})
cloudinary.Api.UrlVideoUp.Transform(new Transformation() .Width(300).Height(200).Crop("fill").Chain() .Overlay("video:dog").Flags("splice").Width(300).Height(200).StartOffset("0").Crop("fill")).BuildVideoTag("kitten_fighting")
To concatenate only a section of a video on to another video use the offset parameters (see Trimming videos for more information on the parameters and their possible values) together with the layer_apply
flag (fl_layer_apply
in URLs). For example, to splice the first 5 seconds of the video named dog
to the end of the same video named dog
rotated by 180 degrees, with both videos set to a width of 300 pixels and a height of 200 pixels.
cl_video_tag("dog", :transformation=>[ {:angle=>180}, {:width=>300, :height=>200, :crop=>"fill"}, {:overlay=>"video:dog", :flags=>"splice", :width=>300, :height=>200, :crop=>"fill"}, {:flags=>"layer_apply", :start_offset=>"0", :duration=>"5"} ])
cl_video_tag("dog", array("transformation"=>array( array("angle"=>180), array("width"=>300, "height"=>200, "crop"=>"fill"), array("overlay"=>"video:dog", "flags"=>"splice", "width"=>300, "height"=>200, "crop"=>"fill"), array("flags"=>"layer_apply", "start_offset"=>"0", "duration"=>"5") )))
CloudinaryVideo("dog").video(transformation=[ {"angle": 180}, {"width": 300, "height": 200, "crop": "fill"}, {"overlay": "video:dog", "flags": "splice", "width": 300, "height": 200, "crop": "fill"}, {"flags": "layer_apply", "start_offset": "0", "duration": "5"} ])
cloudinary.video("dog", {transformation: [ {angle: 180}, {width: 300, height: 200, crop: "fill"}, {overlay: "video:dog", flags: "splice", width: 300, height: 200, crop: "fill"}, {flags: "layer_apply", start_offset: "0", duration: "5"} ]})
cloudinary.url().transformation(new Transformation() .angle(180).chain() .width(300).height(200).crop("fill").chain() .overlay("video:dog").flags("splice").width(300).height(200).crop("fill").chain() .flags("layer_apply").startOffset("0").duration("5")).videoTag("dog")
$.cloudinary.video("dog", {transformation: [ {angle: 180}, {width: 300, height: 200, crop: "fill"}, {overlay: "video:dog", flags: "splice", width: 300, height: 200, crop: "fill"}, {flags: "layer_apply", start_offset: "0", duration: "5"} ]})
cloudinary.Api.UrlVideoUp.Transform(new Transformation() .Angle(180).Chain() .Width(300).Height(200).Crop("fill").Chain() .Overlay("video:dog").Flags("splice").Width(300).Height(200).Crop("fill").Chain() .Flags("layer_apply").StartOffset("0").Duration("5")).BuildVideoTag("dog")
Concatenate videos with images
Cloudinary also supports the concatenation of videos with images by using the following combination of parameters:
overlay
(l
: in URLs) to specify the name of an uploaded image,splice
flag (fl_splice
in URLs) to indicate that the image should be concatenated on to the container video and not added as an overlay,duration
parameter (du
in URLs) to specify the amount of time in seconds to display the image.start_offset
(optional) set to 0 (so_0
in URLs) to concatenate the image at the beginning of the video instead of at the end.layer_apply
flag (fl_layer_apply
) to indicate that the above parameters are applied to the image (with the overlay parameter) and not the containing video.
For example, to concatenate the image named sample
to the start of the video named dog
for a duration of 3 seconds (both the video and image are scaled to a width of 300 pixels and a height of 200 pixels):
cl_video_tag("dog", :transformation=>[ {:width=>300, :height=>200}, {:width=>300, :height=>200, :overlay=>"sample", :flags=>"splice", :duration=>"3"}, {:start_offset=>"0", :flags=>"layer_apply"} ])
cl_video_tag("dog", array("transformation"=>array( array("width"=>300, "height"=>200), array("width"=>300, "height"=>200, "overlay"=>"sample", "flags"=>"splice", "duration"=>"3"), array("start_offset"=>"0", "flags"=>"layer_apply") )))
CloudinaryVideo("dog").video(transformation=[ {"width": 300, "height": 200}, {"width": 300, "height": 200, "overlay": "sample", "flags": "splice", "duration": "3"}, {"start_offset": "0", "flags": "layer_apply"} ])
cloudinary.video("dog", {transformation: [ {width: 300, height: 200}, {width: 300, height: 200, overlay: "sample", flags: "splice", duration: "3"}, {start_offset: "0", flags: "layer_apply"} ]})
cloudinary.url().transformation(new Transformation() .width(300).height(200).chain() .width(300).height(200).overlay("sample").flags("splice").duration("3").chain() .startOffset("0").flags("layer_apply")).videoTag("dog")
$.cloudinary.video("dog", {transformation: [ {width: 300, height: 200}, {width: 300, height: 200, overlay: "sample", flags: "splice", duration: "3"}, {start_offset: "0", flags: "layer_apply"} ]})
cloudinary.Api.UrlVideoUp.Transform(new Transformation() .Width(300).Height(200).Chain() .Width(300).Height(200).Overlay("sample").Flags("splice").Duration("3").Chain() .StartOffset("0").Flags("layer_apply")).BuildVideoTag("dog")
Rotating videos
Rotate the video by any arbitrary angle in degrees with the angle
parameter (a
in URLs). If the angle is not a multiple of 90 then a rectangular bounding box is added containing the rotated video and empty space.
Examples with the uploaded mp4 video named dog
:
Rotating the video by 90 degrees:
Ruby:cl_video_tag("dog", :angle=>90)
PHP:cl_video_tag("dog", array("angle"=>90))
Python:CloudinaryVideo("dog").video(angle=90)
Node.js:cloudinary.video("dog", {angle: 90})
Java:cloudinary.url().transformation(new Transformation().angle(90)).videoTag("dog")
jQuery:$.cloudinary.video("dog", {angle: 90})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().Angle(90)).BuildVideoTag("dog")
Rotating the video by 20 degrees (automatically adds a bounding box):
Ruby:cl_video_tag("dog", :angle=>20)
PHP:cl_video_tag("dog", array("angle"=>20))
Python:CloudinaryVideo("dog").video(angle=20)
Node.js:cloudinary.video("dog", {angle: 20})
Java:cloudinary.url().transformation(new Transformation().angle(20)).videoTag("dog")
jQuery:$.cloudinary.video("dog", {angle: 20})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().Angle(20)).BuildVideoTag("dog")
Rounding corners and creating circular videos
Transforming a video to a rounded version is done using the radius
parameter (r
in URLs) set to the number of pixels the radius of all four corners should be. For example, the uploaded mp4 video named dog
resized to a width of 300 pixels and with rounded corners set to a radius of 20 pixels:
cl_video_tag("dog", :width=>300, :radius=>30)
cl_video_tag("dog", array("width"=>300, "radius"=>30))
CloudinaryVideo("dog").video(width=300, radius=30)
cloudinary.video("dog", {width: 300, radius: 30})
cloudinary.url().transformation(new Transformation().width(300).radius(30)).videoTag("dog")
$.cloudinary.video("dog", {width: 300, radius: 30})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(300).Radius(30)).BuildVideoTag("dog")
Cloudinary also supports cropping videos to the shape of an ellipse. Simply pass max
as the value of the radius
parameter. For example, the uploaded mp4 video named dog
resized to a width of 300 pixels and with maximum radius cropping:
cl_video_tag("dog", :width=>300, :radius=>"max")
cl_video_tag("dog", array("width"=>300, "radius"=>"max"))
CloudinaryVideo("dog").video(width=300, radius="max")
cloudinary.video("dog", {width: 300, radius: "max"})
cloudinary.url().transformation(new Transformation().width(300).radius("max")).videoTag("dog")
$.cloudinary.video("dog", {width: 300, radius: "max"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(300).Radius("max")).BuildVideoTag("dog")
You can also change the background color of the video from the default white color using the background parameter. Likewise, if the width and height of the video are identical (i.e., the same value) then setting the radius parameter to max generates a circular video. For example, the uploaded mp4 video named dog
with width and height set to 300 pixels, and with maximum radius cropping using a blue background:
cl_video_tag("dog", :width=>300, :height=>300, :background=>"blue", :radius=>"max", :crop=>"fill")
cl_video_tag("dog", array("width"=>300, "height"=>300, "background"=>"blue", "radius"=>"max", "crop"=>"fill"))
CloudinaryVideo("dog").video(width=300, height=300, background="blue", radius="max", crop="fill")
cloudinary.video("dog", {width: 300, height: 300, background: "blue", radius: "max", crop: "fill"})
cloudinary.url().transformation(new Transformation().width(300).height(300).background("blue").radius("max").crop("fill")).videoTag("dog")
$.cloudinary.video("dog", {width: 300, height: 300, background: "blue", radius: "max", crop: "fill"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(300).Height(300).Background("blue").Radius("max").Crop("fill")).BuildVideoTag("dog")
The radius parameter can also be used to round the corners of overlays, which are then also given a transparent background. For example, the uploaded mp4 video named dog
overlaid on the same video named dog
with width set to 100 pixels, maximum radius cropping and north east gravity:
cl_video_tag("dog", :transformation=>[ {:width=>300}, {:overlay=>"video:dog", :width=>100, :gravity=>"north_east", :radius=>"max"} ])
cl_video_tag("dog", array("transformation"=>array( array("width"=>300), array("overlay"=>"video:dog", "width"=>100, "gravity"=>"north_east", "radius"=>"max") )))
CloudinaryVideo("dog").video(transformation=[ {"width": 300}, {"overlay": "video:dog", "width": 100, "gravity": "north_east", "radius": "max"} ])
cloudinary.video("dog", {transformation: [ {width: 300}, {overlay: "video:dog", width: 100, gravity: "north_east", radius: "max"} ]})
cloudinary.url().transformation(new Transformation() .width(300).chain() .overlay("video:dog").width(100).gravity("north_east").radius("max")).videoTag("dog")
$.cloudinary.video("dog", {transformation: [ {width: 300}, {overlay: "video:dog", width: 100, gravity: "north_east", radius: "max"} ]})
cloudinary.Api.UrlVideoUp.Transform(new Transformation() .Width(300).Chain() .Overlay("video:dog").Width(100).Gravity("north_east").Radius("max")).BuildVideoTag("dog")
Video settings
The video settings are used for adjusting the video properties of the uploaded file and allow you to manipulate the delivered video file as follows:
- Transcode the video from one format to another.
- Determine the video's quality.
- Control the video bit rate.
- Adjust the video codec settings.
Transcoding videos
Transcoding is the direct conversion of one video encoding format to another. Videos can be uploaded to Cloudinary in a variety of formats: mp4
, webm
, flv
, mov
, ogv
, 3gp
, 3g2
, wmv
, mpeg
, flv
, mkv
or avi
. These formats may not necessarily be best suited for web and mobile usage, so these videos can be dynamically converted and normalized to the relevant web formats, and optimized for different web browsers and mobile devices.
Transcoding the video to another format is done by simply specifying the new format as the file extension of the URL (client libraries can also use the format
parameter to specify the new format). Cloudinary currently supports transcoding videos to one of the following output formats: MP4, OGV, FLV, WebM (see video_codec
in the Video transformations reference table for the default settings for each format). Furthermore, video files can be converted to audio files by stipulating one of the following formats: MP3, OGG, WAV.
For example, transcoding the format of the uploaded mp4 video named dog
to WebM format by changing the file extension to .webm
:
cl_video_tag("dog")
cl_video_tag("dog")
CloudinaryVideo("dog").video()
cloudinary.video("dog")
cloudinary.url().videoTag("dog")
$.cloudinary.video("dog")
cloudinary.Api.UrlVideoUp.BuildVideoTag("dog")
cloudinary_url("dog.webm", :resource_type => :video)
Which is equivalent to:
cloudinary_url("dog", :resource_type => :video, :format => "webm").
Another way to transcode the format of an uploaded video is to use the format
parameter (f
for URLs).
For example, transcoding the uploaded mp4 video named dog
to WebM with a parameter:
cl_video_tag("dog", :format=>"webm")
cl_video_tag("dog", array("format"=>"webm"))
CloudinaryVideo("dog").video(format="webm")
cloudinary.video("dog", {format: "webm"})
cloudinary.url().format("webm").videoTag("dog")
$.cloudinary.video("dog", {format: "webm"})
cloudinary.Api.UrlVideoUp.Format("webm").BuildVideoTag("dog")
Quality control
Control the video quality with the quality
parameter (q
in URLs). This parameter represents a mapping between the actual low-level settings of each different video format normalized to a quality value between 1 (lowest) and 100 (highest). Reducing the quality is a tradeoff between visual quality and file size. See video_codec
in the Video transformations reference table for the default settings for each format.
For example, reducing the quality of the uploaded mp4 video named dog
to 50 results in a file size of 1.1 MB compared to the original file size of 9.8MB:
cl_video_tag("dog", :quality=>50)
cl_video_tag("dog", array("quality"=>50))
CloudinaryVideo("dog").video(quality=50)
cloudinary.video("dog", {quality: 50})
cloudinary.url().transformation(new Transformation().quality(50)).videoTag("dog")
$.cloudinary.video("dog", {quality: 50})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Quality(50)).BuildVideoTag("dog")
Bit rate control
Use the bit_rate
parameter (br
in URLs) for advanced control of the video bit rate. This parameter controls the number of bits used to represent the video data. The higher the bit rate, the higher the visual quality of the video, but the larger the file size as well. Bit rate can take one of the following values:
- An integer e.g.
120000
. - A string supporting ?k’ and ?m’ (kilobits and megabits respectively) e.g.
500k
or2m
.
For example, changing the bit rate of the uploaded mp4 video named dog
to 500 kilobits (also reducing file size down to 1MB):
cl_video_tag("dog", :bit_rate=>"250k")
cl_video_tag("dog", array("bit_rate"=>"250k"))
CloudinaryVideo("dog").video(bit_rate="250k")
cloudinary.video("dog", {bit_rate: "250k"})
cloudinary.url().transformation(new Transformation().bitRate("250k")).videoTag("dog")
$.cloudinary.video("dog", {bit_rate: "250k"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().BitRate("250k")).BuildVideoTag("dog")
Video codec settings
The video_codec
parameter (vc
in URLs) is used to determine the video codec, video profile and level to use in the form <codec>[:<profile>:[<level>]]
e.g. vc_h264:baseline:3.1
or vc_h264
or vc_h264:high
. You can set this parameter to auto
instead, to normalize and optimize the video for web (including audio settings).
By default, any transformation applied to the video (e.g., resizing) implicitly also uses the auto settings when delivering the transformed video, so using the auto setting is only needed when delivering the same format as originally uploaded with optimization but without any additional transformations. See video_codec
in the Video transformations reference table for the default settings for each format.
Examples with the uploaded mp4 video named dog
:
Setting the video codec to h264, the profile to baseline and the level to 3.1:
Ruby:cl_video_tag("dog", :video_codec=>"h264:baseline:3.1")
PHP:cl_video_tag("dog", array("video_codec"=>"h264:baseline:3.1"))
Python:CloudinaryVideo("dog").video(video_codec="h264:baseline:3.1")
Node.js:cloudinary.video("dog", {video_codec: "h264:baseline:3.1"})
Java:cloudinary.url().transformation(new Transformation().videoCodec("h264:baseline:3.1")).videoTag("dog")
jQuery:$.cloudinary.video("dog", {video_codec: "h264:baseline:3.1"})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().VideoCodec("h264:baseline:3.1")).BuildVideoTag("dog")
Normalizing the video for web with the default settings:
Ruby:cl_video_tag("dog", :video_codec=>"auto")
PHP:cl_video_tag("dog", array("video_codec"=>"auto"))
Python:CloudinaryVideo("dog").video(video_codec="auto")
Node.js:cloudinary.video("dog", {video_codec: "auto"})
Java:cloudinary.url().transformation(new Transformation().videoCodec("auto")).videoTag("dog")
jQuery:$.cloudinary.video("dog", {video_codec: "auto"})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().VideoCodec("auto")).BuildVideoTag("dog")
Notes:
Currently supported for
vp9
,h264
andh265
. Only theh264
codec (mp4
) has profile or level parameters, with profile:baseline
,main
orhigh
and level:3.0
,3.1
,4.0
,4.1
or4.2
.Client libraries can optionally enter the parameter as a structure. For example, in Ruby:
video_codec: { codec: 'h264', profile: 'baseline', level: '3.1' }
Video Effects
Video effects can be applied to an uploaded video file and allow you to manipulate the delivered video file as follows:
- Change the video playback speed using accelerate.
- Add visual noise.
- Remove small motion shifts with deshake.
- Fade in to or fade out of a video.
- Calibrate the contrast.
- Control the brightness.
- Balance the saturation.
- Regulate the gamma.
- Apply a vignette effect.
- Adjust the volume.
- Play the video or audio in reverse
Change video playback speed
Use the accelerate
video effect (e_accelerate
in URLs) to either speed up or slow down the video playback speed. The effect accepts an integer value between -50 and 100 that determines how to change the playback speed (in percent), with a positive value for speeding up the video, and a negative value for slowing down the video.
For example, the uploaded mp4 video named dog
resized to a width of 300 pixels and accelerated by 100% (twice normal speed):
cl_video_tag("dog", :width=>300, :effect=>"accelerate:100")
cl_video_tag("dog", array("width"=>300, "effect"=>"accelerate:100"))
CloudinaryVideo("dog").video(width=300, effect="accelerate:100")
cloudinary.video("dog", {width: 300, effect: "accelerate:100"})
cloudinary.url().transformation(new Transformation().width(300).effect("accelerate:100")).videoTag("dog")
$.cloudinary.video("dog", {width: 300, effect: "accelerate:100"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(300).Effect("accelerate:100")).BuildVideoTag("dog")
Add visual noise
Use the noise
video effect (e_noise
in URLs) to add visual noise to a video. Noise is a random pattern superimposed on the video, visible as a random flicker of "dots" or "snow". The amount of noise added to the video is set by adding an integer value to the parameter, ranging from 0 (no noise) up to 100.
For example, applying the noise effect with a value of 50 to the mp4 video named dog
, resized to a width of 300 pixels:
cl_video_tag("dog", :width=>300, :effect=>"noise:50")
cl_video_tag("dog", array("width"=>300, "effect"=>"noise:50"))
CloudinaryVideo("dog").video(width=300, effect="noise:50")
cloudinary.video("dog", {width: 300, effect: "noise:50"})
cloudinary.url().transformation(new Transformation().width(300).effect("noise:50")).videoTag("dog")
$.cloudinary.video("dog", {width: 300, effect: "noise:50"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(300).Effect("noise:50")).BuildVideoTag("dog")
Remove small motion shifts
The deshake
video effect (e_deshake
in URLs) applies a filter that attempts to fix small changes in horizontal and vertical motion shift. This filter helps remove camera shake from hand-holding a camera, bumping a tripod, moving on a vehicle, etc. A value between 0-64 can also be specified that determines the maximum extent of movement in the horizontal and/or vertical direction in pixels, with the default set to 16 pixels.
For example, applying the deshake effect with a maximum motion shift of 32 pixels to the mp4 video named dog
:
cl_video_tag("dog", :effect=>"deshake:32")
cl_video_tag("dog", array("effect"=>"deshake:32"))
CloudinaryVideo("dog").video(effect="deshake:32")
cloudinary.video("dog", {effect: "deshake:32"})
cloudinary.url().transformation(new Transformation().effect("deshake:32")).videoTag("dog")
$.cloudinary.video("dog", {effect: "deshake:32"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Effect("deshake:32")).BuildVideoTag("dog")
Fade in and out
Use the fade
video effect (e_fade
in URLs) to either fade in at the beginning of a video or fade out at the end. The effect accepts a parameter that determines the duration of the fade effect in milliseconds, with a positive value for fading in at the beginning of the video, and a negative value for fading out at the end of the video. The fade effect can also be chained to allow for both fading in and then fading out of a video.
For example, applying the fade effect to the mp4 video named dog
, first by fading in at the beginning of the video over 2 seconds and then fading out at the end of the video over 4 seconds:
cl_video_tag("dog", :transformation=>[ {:effect=>"fade:2000"}, {:effect=>"fade:-4000"} ])
cl_video_tag("dog", array("transformation"=>array( array("effect"=>"fade:2000"), array("effect"=>"fade:-4000") )))
CloudinaryVideo("dog").video(transformation=[ {"effect": "fade:2000"}, {"effect": "fade:-4000"} ])
cloudinary.video("dog", {transformation: [ {effect: "fade:2000"}, {effect: "fade:-4000"} ]})
cloudinary.url().transformation(new Transformation() .effect("fade:2000").chain() .effect("fade:-4000")).videoTag("dog")
$.cloudinary.video("dog", {transformation: [ {effect: "fade:2000"}, {effect: "fade:-4000"} ]})
cloudinary.Api.UrlVideoUp.Transform(new Transformation() .Effect("fade:2000").Chain() .Effect("fade:-4000")).BuildVideoTag("dog")
The fade
effect is also useful for fading in and out of video overlays. For example, adding an overlay of kitten_fighting
scaled to a width of 100 pixels to the mp4 video named dog
, that fades in over 3 seconds:
cl_video_tag("dog", :overlay=>"video:kitten_fighting", :effect=>"fade:3000", :width=>100)
cl_video_tag("dog", array("overlay"=>"video:kitten_fighting", "effect"=>"fade:3000", "width"=>100))
CloudinaryVideo("dog").video(overlay="video:kitten_fighting", effect="fade:3000", width=100)
cloudinary.video("dog", {overlay: "video:kitten_fighting", effect: "fade:3000", width: 100})
cloudinary.url().transformation(new Transformation().overlay("video:kitten_fighting").effect("fade:3000").width(100)).videoTag("dog")
$.cloudinary.video("dog", {overlay: "video:kitten_fighting", effect: "fade:3000", width: 100})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Overlay("video:kitten_fighting").Effect("fade:3000").Width(100)).BuildVideoTag("dog")
Calibrate contrast
Use the contrast
video effect (e_contrast
in URLs) to either increase or decrease the contrast level in a video. Contrast is determined by the difference in brightness and color that makes objects within the video distinguishable from other objects within the same video. The contrast level can be decreased down to -300 or increased up to +100, with a default level of 0.
For example, applying the contrast effect to the mp4 video named dog
, by increasing the contrast level by 50:
cl_video_tag("dog", :width=>500, :effect=>"contrast:50")
cl_video_tag("dog", array("width"=>500, "effect"=>"contrast:50"))
CloudinaryVideo("dog").video(width=500, effect="contrast:50")
cloudinary.video("dog", {width: 500, effect: "contrast:50"})
cloudinary.url().transformation(new Transformation().width(500).effect("contrast:50")).videoTag("dog")
$.cloudinary.video("dog", {width: 500, effect: "contrast:50"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(500).Effect("contrast:50")).BuildVideoTag("dog")
Control brightness
Use the brightness
video effect (e_brightness
in URLs) to either increase or decrease the brightness level in a video. Brightness determines how much the objects in a video appear to be radiating light. The brightness level can be decreased down to -100 or increased up to +100, with a default level of 0.
For example, applying the brightness effect to the mp4 video named dog
, by increasing the brightness level by 30:
cl_video_tag("dog", :width=>500, :effect=>"brightness:30")
cl_video_tag("dog", array("width"=>500, "effect"=>"brightness:30"))
CloudinaryVideo("dog").video(width=500, effect="brightness:30")
cloudinary.video("dog", {width: 500, effect: "brightness:30"})
cloudinary.url().transformation(new Transformation().width(500).effect("brightness:30")).videoTag("dog")
$.cloudinary.video("dog", {width: 500, effect: "brightness:30"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(500).Effect("brightness:30")).BuildVideoTag("dog")
Balance saturation
Use the saturation
video effect (e_saturation
in URLs) to either increase or decrease the saturation level in a video. Saturation determines the colorfulness of objects relative to their brightness. The saturation level can be decreased down to -200 or increased up to +100, with a default level of 0.
For example, applying the saturation effect to the mp4 video named dog
, by decreasing the saturation level by -50:
cl_video_tag("dog", :width=>500, :effect=>"saturation:-50")
cl_video_tag("dog", array("width"=>500, "effect"=>"saturation:-50"))
CloudinaryVideo("dog").video(width=500, effect="saturation:-50")
cloudinary.video("dog", {width: 500, effect: "saturation:-50"})
cloudinary.url().transformation(new Transformation().width(500).effect("saturation:-50")).videoTag("dog")
$.cloudinary.video("dog", {width: 500, effect: "saturation:-50"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(500).Effect("saturation:-50")).BuildVideoTag("dog")
Regulate gamma
Use the gamma
video effect (e_gamma
in URLs) to either increase or decrease the gamma level in a video. Gamma determines the relative luminance of objects within the video. The gamma level can be decreased down to a -100 or increased up to +100, with a default level of 0.
For example, applying the gamma effect to the mp4 video named dog
, by decreasing the gamma level to -20:
cl_video_tag("dog", :width=>500, :effect=>"gamma:-20")
cl_video_tag("dog", array("width"=>500, "effect"=>"gamma:-20"))
CloudinaryVideo("dog").video(width=500, effect="gamma:-20")
cloudinary.video("dog", {width: 500, effect: "gamma:-20"})
cloudinary.url().transformation(new Transformation().width(500).effect("gamma:-20")).videoTag("dog")
$.cloudinary.video("dog", {width: 500, effect: "gamma:-20"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(500).Effect("gamma:-20")).BuildVideoTag("dog")
Apply vignette
Use the vignette
video effect (e_vignette
in URLs) to apply a vignette filter to a video. The vignette filter creates an effect where the video is clear in the center and fades out towards the edges. The vignette effect parameter accepts a value between 0 and 100 to determine how quickly the video fades out from a clear center.
For example, applying the vignette effect with a level of 50 to the mp4 video named dog
:
cl_video_tag("dog", :width=>500, :effect=>"vignette:50")
cl_video_tag("dog", array("width"=>500, "effect"=>"vignette:50"))
CloudinaryVideo("dog").video(width=500, effect="vignette:50")
cloudinary.video("dog", {width: 500, effect: "vignette:50"})
cloudinary.url().transformation(new Transformation().width(500).effect("vignette:50")).videoTag("dog")
$.cloudinary.video("dog", {width: 500, effect: "vignette:50"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(500).Effect("vignette:50")).BuildVideoTag("dog")
Adjust volume
Control the audio volume of a video with the volume
effect parameter (e_volume
in URLs). This parameter represents a percentage of the original volume and can take a value between -100 (no audio) to +400, with a default of 0.
For example, increasing the volume in the mp4 video named dog
by 50%:
cl_video_tag("dog", :width=>500, :effect=>"volume:50")
cl_video_tag("dog", array("width"=>500, "effect"=>"volume:50"))
CloudinaryVideo("dog").video(width=500, effect="volume:50")
cloudinary.video("dog", {width: 500, effect: "volume:50"})
cloudinary.url().transformation(new Transformation().width(500).effect("volume:50")).videoTag("dog")
$.cloudinary.video("dog", {width: 500, effect: "volume:50"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(500).Effect("volume:50")).BuildVideoTag("dog")
Reverse playback
Use the reverse
effect (e_reverse
in URLs) to play a video or audio file backwards.
For example, in the following reversed video, you can watch a woman un-sketch her phone design:
cl_video_tag("pencil_sketch", :width=>500, :effect=>"reverse")
cl_video_tag("pencil_sketch", array("width"=>500, "effect"=>"reverse"))
CloudinaryVideo("pencil_sketch").video(width=500, effect="reverse")
cloudinary.video("pencil_sketch", {width: 500, effect: "reverse"})
cloudinary.url().transformation(new Transformation().width(500).effect("reverse")).videoTag("pencil_sketch")
$.cloudinary.video("pencil_sketch", {width: 500, effect: "reverse"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(500).Effect("reverse")).BuildVideoTag("pencil_sketch")
Tip: Play the video in both directions by splicing a reversed version of the video after the original.
Audio settings
The audio settings are used for adjusting the audio properties of the file and allow you to manipulate the delivered video file as follows:
- Control the audio sampling frequency.
- Select the audio codec (or remove the audio).
Audio frequency control
Use the audio_frequency
parameter (af
in URLs) to control the audio sampling frequency. This parameter represents an integer value in Hz. See audio_frequency
in the Video transformations reference table for the possible values.
For example, setting the audio frequency to 22050 Hz in the uploaded mp4 video named dog
:
cl_video_tag("dog", :audio_frequency=>"22050")
cl_video_tag("dog", array("audio_frequency"=>"22050"))
CloudinaryVideo("dog").video(audio_frequency="22050")
cloudinary.video("dog", {audio_frequency: "22050"})
cloudinary.url().transformation(new Transformation().audioFrequency("22050")).videoTag("dog")
$.cloudinary.video("dog", {audio_frequency: "22050"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().AudioFrequency("22050")).BuildVideoTag("dog")
Audio codec settings
Use the audio_codec
parameter (ac
in URLs) to set the audio codec or remove the audio channel completely as follows:
none
removes the audio channelaac
(mp4 or flv only)vorbis
(ogv or webm only)mp3
(mp4 or flv only)
For example, removing the audio channel from the uploaded mp4 video named dog
:
cl_video_tag("dog", :audio_codec=>"none")
cl_video_tag("dog", array("audio_codec"=>"none"))
CloudinaryVideo("dog").video(audio_codec="none")
cloudinary.video("dog", {audio_codec: "none"})
cloudinary.url().transformation(new Transformation().audioCodec("none")).videoTag("dog")
$.cloudinary.video("dog", {audio_codec: "none"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().AudioCodec("none")).BuildVideoTag("dog")
Generating video thumbnails
Cloudinary makes it easy to deliver image thumbnails of any frame within an uploaded video by simply specifying the image format needed as the file extension of the URL (e.g. jpg, png, webp, wdp).
For example, generating a JPG image thumbnail URL of the uploaded mp4 video named dog
:
cl_image_tag("dog.jpg", :resource_type=>"video")
cl_image_tag("dog.jpg", array("resource_type"=>"video"))
CloudinaryVideo("dog.jpg").image()
cloudinary.image("dog.jpg", {resource_type: "video"})
cloudinary.url().resourceType("video").imageTag("dog.jpg")
$.cloudinary.image("dog.jpg", {resource_type: "video"})
cloudinary.Api.UrlVideoUp.BuildImageTag("dog.jpg")

To control which frame is captured from the video as a thumbnail (the middle frame is selected by default), use the start_offset
parameter (so
in URLs) with one of the following values:
- A float representing the time in seconds from the beginning of the video e.g.
5.44
. - A string representing the percentage of the video from the beginning. This string consists of a number with a
p
appended e.g.35p
(0p is the first frame and 100p is the last frame). The client libraries also support appending a%
instead of ap
. - A value of
auto
which automatically selects a frame that best matches the average value of a color distribution analysis of the first few seconds of the video, making it more likely to display a typical frame.
For example, generating a JPG image thumbnail of the frame at 8.5 seconds in the uploaded mp4 video named dog
:
cl_image_tag("dog.jpg", :start_offset=>"8.5", :resource_type=>"video")
cl_image_tag("dog.jpg", array("start_offset"=>"8.5", "resource_type"=>"video"))
CloudinaryVideo("dog.jpg").image(start_offset="8.5")
cloudinary.image("dog.jpg", {start_offset: "8.5", resource_type: "video"})
cloudinary.url().transformation(new Transformation().startOffset("8.5")).resourceType("video").imageTag("dog.jpg")
$.cloudinary.image("dog.jpg", {start_offset: "8.5", resource_type: "video"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().StartOffset("8.5")).BuildImageTag("dog.jpg")
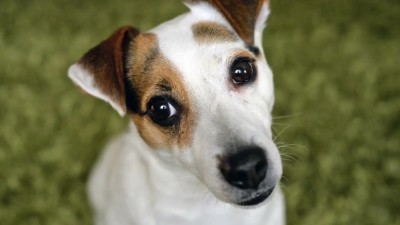
Once generated, the thumbnail image supports further manipulation as any other image (see image transformations for more details).
For example, generating a cropped JPG image thumbnail with a width and height of 350 pixels, of the frame at 4 seconds in the uploaded mp4 video named dog
. The image is also rendered in gray scale, with rounded corners and a 5 pixel wide black border:
cl_image_tag("dog.jpg", :start_offset=>"4", :width=>350, :height=>350, :radius=>20, :effect=>"grayscale", :border=>"5px_solid_black", :crop=>"crop", :resource_type=>"video")
cl_image_tag("dog.jpg", array("start_offset"=>"4", "width"=>350, "height"=>350, "radius"=>20, "effect"=>"grayscale", "border"=>"5px_solid_black", "crop"=>"crop", "resource_type"=>"video"))
CloudinaryVideo("dog.jpg").image(start_offset="4", width=350, height=350, radius=20, effect="grayscale", border="5px_solid_black", crop="crop")
cloudinary.image("dog.jpg", {start_offset: "4", width: 350, height: 350, radius: 20, effect: "grayscale", border: "5px_solid_black", crop: "crop", resource_type: "video"})
cloudinary.url().transformation(new Transformation().startOffset("4").width(350).height(350).radius(20).effect("grayscale").border("5px_solid_black").crop("crop")).resourceType("video").imageTag("dog.jpg")
$.cloudinary.image("dog.jpg", {start_offset: "4", width: 350, height: 350, radius: 20, effect: "grayscale", border: "5px_solid_black", crop: "crop", resource_type: "video"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().StartOffset("4").Width(350).Height(350).Radius(20).Effect("grayscale").Border("5px_solid_black").Crop("crop")).BuildImageTag("dog.jpg")

Generate waveform images
You can create audio waveform images from audio or video files just as easily as you can create thumbnails for images and videos, with fine control over the look & feel of the generated waveform image.
This section is divided into the following subsections:
Creating waveform images
To create a waveform image from an audio or video file uploaded to your Cloudinary account, change the file extension (format) of the Cloudinary delivery URL to any image format (e.g., PNG) and enable the waveform
flag (fl_waveform
in URLs). By default, the resulting waveform image is delivered with a very high resolution, so you will probably also want to scale down the resulting image.
For example, to generate a PNG waveform image from the audio track of the dog.mp4
video file uploaded to Cloudinary's demo account, scaled to a height of 200 pixels and a width of 500 pixels:
cl_image_tag("dog.png", :height=>200, :width=>500, :flags=>"waveform", :resource_type=>"video")
cl_image_tag("dog.png", array("height"=>200, "width"=>500, "flags"=>"waveform", "resource_type"=>"video"))
CloudinaryVideo("dog.png").image(height=200, width=500, flags="waveform")
cloudinary.image("dog.png", {height: 200, width: 500, flags: "waveform", resource_type: "video"})
cloudinary.url().transformation(new Transformation().height(200).width(500).flags("waveform")).resourceType("video").imageTag("dog.png")
$.cloudinary.image("dog.png", {height: 200, width: 500, flags: "waveform", resource_type: "video"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Height(200).Width(500).Flags("waveform")).BuildImageTag("dog.png")

Customizing the waveform image
You can also control the colors used in the waveform image with the color
parameter (co
in URLs) to set the color for the waveform (default white
) and the background
parameter (b
in URLs) to set the background color of the image (default black
).
For example, to generate the same PNG waveform image from the audio track of the dog.mp4
video file in the example above in inverted colors - with the waveform rendered in black on a white background:
cl_image_tag("dog.png", :height=>200, :width=>500, :flags=>"waveform", :color=>"black", :background=>"white", :resource_type=>"video")
cl_image_tag("dog.png", array("height"=>200, "width"=>500, "flags"=>"waveform", "color"=>"black", "background"=>"white", "resource_type"=>"video"))
CloudinaryVideo("dog.png").image(height=200, width=500, flags="waveform", color="black", background="white")
cloudinary.image("dog.png", {height: 200, width: 500, flags: "waveform", color: "black", background: "white", resource_type: "video"})
cloudinary.url().transformation(new Transformation().height(200).width(500).flags("waveform").color("black").background("white")).resourceType("video").imageTag("dog.png")
$.cloudinary.image("dog.png", {height: 200, width: 500, flags: "waveform", color: "black", background: "white", resource_type: "video"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Height(200).Width(500).Flags("waveform").Color("black").Background("white")).BuildImageTag("dog.png")

If you want to capture the waveform of a specific segment of the audio file, you can select from the following parameters to specify the section of the file to sample for the waveform:
start_offset
(so
in URLs) specifies the start of the sample.end_offset
(eo
in URLs) specifies the end of the sample.duration
(du
in URLs) specifies the duration of the sample.
For example, to display a PNG waveform image of a sample from the 2 second mark until the 4 second mark of the dog.mp4
video file uploaded to Cloudinary's demo account, scaled to a height of 250 pixels and a width of 400 pixels, with the waveform rendered in blue on a green background:
cl_image_tag("dog.png", :height=>250, :width=>400, :flags=>"waveform", :start_offset=>"2", :end_offset=>"4", :color=>"blue", :background=>"#02b30a", :resource_type=>"video")
cl_image_tag("dog.png", array("height"=>250, "width"=>400, "flags"=>"waveform", "start_offset"=>"2", "end_offset"=>"4", "color"=>"blue", "background"=>"#02b30a", "resource_type"=>"video"))
CloudinaryVideo("dog.png").image(height=250, width=400, flags="waveform", start_offset="2", end_offset="4", color="blue", background="#02b30a")
cloudinary.image("dog.png", {height: 250, width: 400, flags: "waveform", start_offset: "2", end_offset: "4", color: "blue", background: "#02b30a", resource_type: "video"})
cloudinary.url().transformation(new Transformation().height(250).width(400).flags("waveform").startOffset("2").endOffset("4").color("blue").background("#02b30a")).resourceType("video").imageTag("dog.png")
$.cloudinary.image("dog.png", {height: 250, width: 400, flags: "waveform", start_offset: "2", end_offset: "4", color: "blue", background: "#02b30a", resource_type: "video"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Height(250).Width(400).Flags("waveform").StartOffset("2").EndOffset("4").Color("blue").Background("#02b30a")).BuildImageTag("dog.png")

The generated image waveforms can be further manipulated to match any graphic design and any responsive layout, just like any other image uploaded to Cloudinary.
Adding image overlays
Add an overlay image over the video with the overlay
parameter (l
in URLs) and the public ID of a previously uploaded PNG image (e.g. l_watermark
for an image with the public ID of watermark
). You can determine the dimension and position of the overlay using the width, height, x, y and gravity parameters the same way as used for images (see Adding watermarks, credits, badges and text overlays to images for more details). Furthermore, it is possible to control when the overlay is displayed by using any combination of the 3 offset parameters (see Trimming videos for more information on the parameters and their possible values). The overlay can also be further manipulated like any other image uploaded to Cloudinary
For example, adding an overlay of a PNG image called cloudinary_icon
to the mp4 video named dog
, that appears after 6.5 seconds and disappears at the 10 second mark. The overlay is also made into a watermark by reducing the opacity to 50 using the o
parameter and increasing the brightness to 200% using the e_brightness
effect:
cl_video_tag("dog", :overlay=>"cloudinary_icon", :start_offset=>"6.5", :end_offset=>"10", :opacity=>50, :effect=>"brightness:200")
cl_video_tag("dog", array("overlay"=>"cloudinary_icon", "start_offset"=>"6.5", "end_offset"=>"10", "opacity"=>50, "effect"=>"brightness:200"))
CloudinaryVideo("dog").video(overlay="cloudinary_icon", start_offset="6.5", end_offset="10", opacity=50, effect="brightness:200")
cloudinary.video("dog", {overlay: "cloudinary_icon", start_offset: "6.5", end_offset: "10", opacity: 50, effect: "brightness:200"})
cloudinary.url().transformation(new Transformation().overlay("cloudinary_icon").startOffset("6.5").endOffset("10").opacity(50).effect("brightness:200")).videoTag("dog")
$.cloudinary.video("dog", {overlay: "cloudinary_icon", start_offset: "6.5", end_offset: "10", opacity: 50, effect: "brightness:200"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Overlay("cloudinary_icon").StartOffset("6.5").EndOffset("10").Opacity(50).Effect("brightness:200")).BuildVideoTag("dog")
Adding video overlays
Add another video as an overlay over the container video by using the overlay video parameter (l_video:
in URLs) and the public ID of a previously uploaded video (e.g. l_video:dog
for a video with the public ID of dog
). You can determine the dimension and position of the video overlay using the width, height, x, y and gravity parameters the same way as used for images (see Adding image overlays for more details). Furthermore, it is possible to control when the video overlay is displayed by using any combination of the 3 offset parameters (see Trimming videos for more information on the parameters and their possible values). The overlay can also be further manipulated like any other video uploaded to Cloudinary.
For example, adding an overlay of a video named dog
to the same mp4 video named dog
, that appears after 4.5 seconds and disappears at the 8 second mark. The overlay is also rotated by 90 degrees, set with a gravity of 'east' and scaled to a width of 200 pixels.
cl_video_tag("dog", :overlay=>"video:dog", :start_offset=>"4.5", :end_offset=>"8", :angle=>90, :width=>200, :gravity=>"east")
cl_video_tag("dog", array("overlay"=>"video:dog", "start_offset"=>"4.5", "end_offset"=>"8", "angle"=>90, "width"=>200, "gravity"=>"east"))
CloudinaryVideo("dog").video(overlay="video:dog", start_offset="4.5", end_offset="8", angle=90, width=200, gravity="east")
cloudinary.video("dog", {overlay: "video:dog", start_offset: "4.5", end_offset: "8", angle: 90, width: 200, gravity: "east"})
cloudinary.url().transformation(new Transformation().overlay("video:dog").startOffset("4.5").endOffset("8").angle(90).width(200).gravity("east")).videoTag("dog")
$.cloudinary.video("dog", {overlay: "video:dog", start_offset: "4.5", end_offset: "8", angle: 90, width: 200, gravity: "east"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Overlay("video:dog").StartOffset("4.5").EndOffset("8").Angle(90).Width(200).Gravity("east")).BuildVideoTag("dog")
Adding text captions
Add a text caption over the video with the text:
property of the overlay
parameter ( l_text:
in URLs), followed by the font name and size (separated with an underscore), a colon, and then the text string to display. For example, to overlay the text string "Sample Video" in the Arial font with a size of 40 pixels:l_text:arial_40:Sample Video
.
As for displaying image overlays, you can also determine the dimension and position of the text caption using the width, height, x, y and gravity parameters and control when the caption is displayed by using any combination of the 3 offset parameters (see Trimming videos for more information on the parameters and their possible values). The resulting caption is actually an image created on the fly and can be further manipulated like any other image uploaded to Cloudinary.
For example, adding the text string "Cool Video" in 60 pt Arial font at a distance of 80 pixels from the bottom of the mp4 video named dog
, that appears after 2 seconds and disappears at the 5 second mark:
cl_video_tag("dog", :overlay=>"text:arial_60:Cool%20Video", :gravity=>"south", :y=>80, :start_offset=>"2", :end_offset=>"5")
cl_video_tag("dog", array("overlay"=>"text:arial_60:Cool%20Video", "gravity"=>"south", "y"=>80, "start_offset"=>"2", "end_offset"=>"5"))
CloudinaryVideo("dog").video(overlay="text:arial_60:Cool%20Video", gravity="south", y=80, start_offset="2", end_offset="5")
cloudinary.video("dog", {overlay: "text:arial_60:Cool%20Video", gravity: "south", y: 80, start_offset: "2", end_offset: "5"})
cloudinary.url().transformation(new Transformation().overlay("text:arial_60:Cool%20Video").gravity("south").y(80).startOffset("2").endOffset("5")).videoTag("dog")
$.cloudinary.video("dog", {overlay: "text:arial_60:Cool%20Video", gravity: "south", y: 80, start_offset: "2", end_offset: "5"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Overlay("text:arial_60:Cool%20Video").Gravity("south").Y(80).StartOffset("2").EndOffset("5")).BuildVideoTag("dog")
Multiple overlays can easily be added to a video. The following example adds both an image overlay and a text caption to the mp4 video named dog
as follows:
- An overlay of a PNG image called
cloudinary_icon
with a width of 200 pixels on the east side of the video, that appears after 1 second, and disappears at the 11 second mark. - The text string "Sample Video" in 40 pt Arial font at a distance of 60 pixels from the bottom of the video, that appears after 2 seconds.
Ruby:
cl_video_tag("dog", :transformation=>[ {:overlay=>"cloudinary_icon", :width=>200, :gravity=>"east", :start_offset=>"1", :end_offset=>"11"}, {:overlay=>"text:arial_40:Sample%20Video", :gravity=>"south", :y=>60, :start_offset=>"2"} ])
PHP:cl_video_tag("dog", array("transformation"=>array( array("overlay"=>"cloudinary_icon", "width"=>200, "gravity"=>"east", "start_offset"=>"1", "end_offset"=>"11"), array("overlay"=>"text:arial_40:Sample%20Video", "gravity"=>"south", "y"=>60, "start_offset"=>"2") )))
Python:CloudinaryVideo("dog").video(transformation=[ {"overlay": "cloudinary_icon", "width": 200, "gravity": "east", "start_offset": "1", "end_offset": "11"}, {"overlay": "text:arial_40:Sample%20Video", "gravity": "south", "y": 60, "start_offset": "2"} ])
Node.js:cloudinary.video("dog", {transformation: [ {overlay: "cloudinary_icon", width: 200, gravity: "east", start_offset: "1", end_offset: "11"}, {overlay: "text:arial_40:Sample%20Video", gravity: "south", y: 60, start_offset: "2"} ]})
Java:cloudinary.url().transformation(new Transformation() .overlay("cloudinary_icon").width(200).gravity("east").startOffset("1").endOffset("11").chain() .overlay("text:arial_40:Sample%20Video").gravity("south").y(60).startOffset("2")).videoTag("dog")
jQuery:$.cloudinary.video("dog", {transformation: [ {overlay: "cloudinary_icon", width: 200, gravity: "east", start_offset: "1", end_offset: "11"}, {overlay: "text:arial_40:Sample%20Video", gravity: "south", y: 60, start_offset: "2"} ]})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation() .Overlay("cloudinary_icon").Width(200).Gravity("east").StartOffset("1").EndOffset("11").Chain() .Overlay("text:arial_40:Sample%20Video").Gravity("south").Y(60).StartOffset("2")).BuildVideoTag("dog")
Adding subtitles
You can add subtitles to videos from SRT files previously uploaded to Cloudinary as raw files with the subtitles:
property of the overlay
parameter ( l_subtitles:
in URLs), followed by the SRT public ID (including the .srt extension).
For example, to overlay the subtitles in the sample_sub_en.srt
file to the mp4 video named dog
:
cl_video_tag("dog", :overlay=>"subtitles:sample_sub_en.srt")
cl_video_tag("dog", array("overlay"=>"subtitles:sample_sub_en.srt"))
CloudinaryVideo("dog").video(overlay="subtitles:sample_sub_en.srt")
cloudinary.video("dog", {overlay: "subtitles:sample_sub_en.srt"})
cloudinary.url().transformation(new Transformation().overlay("subtitles:sample_sub_en.srt")).videoTag("dog")
$.cloudinary.video("dog", {overlay: "subtitles:sample_sub_en.srt"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Overlay("subtitles:sample_sub_en.srt")).BuildVideoTag("dog")
You can also optionally include font and size settings (separated with an underscore) before the subtitles file name. For example, to overlay the subtitles in the sample_sub_en.srt
file to the mp4 video named dog
, using the Arial font with a size of 20 pixels:
cl_video_tag("dog", :overlay=>"subtitles:arial_20:sample_sub_en.srt")
cl_video_tag("dog", array("overlay"=>"subtitles:arial_20:sample_sub_en.srt"))
CloudinaryVideo("dog").video(overlay="subtitles:arial_20:sample_sub_en.srt")
cloudinary.video("dog", {overlay: "subtitles:arial_20:sample_sub_en.srt"})
cloudinary.url().transformation(new Transformation().overlay("subtitles:arial_20:sample_sub_en.srt")).videoTag("dog")
$.cloudinary.video("dog", {overlay: "subtitles:arial_20:sample_sub_en.srt"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Overlay("subtitles:arial_20:sample_sub_en.srt")).BuildVideoTag("dog")
You can also control the color of the text by adding the color
property (co
in URLs) to change the fill color of the text, and adding the background
property (b
in URLs) to change the color of the text border. Colors can be set as an RGB hex triplet (e.g. b_rgb:3e2222
), a 3 character RGB hex (e.g. co_rgb:777
) or a named color (e.g. b_green
). You can also use a RGB hex quadruplet, where the 4th value represents the opacity (e.g. b_rgb:3e222266
). Cloudinary's client libraries also support a #
shortcut for RGB (e.g. setting the color to #3e2222 which is then translated to b_rgb:3e2222
). By default the text has a black border with a white fill.
For example, to overlay the subtitles in the sample_sub_en.srt
file on to the mp4 video named dog
, using the Arial font with a size of 75 pixels, in 50% transparent yellow text (FFFF0080) with a black border:
cl_video_tag("dog", :overlay=>"subtitles:arial_75:sample_sub_en.srt", :color=>"#ffff0080", :background=>"black")
cl_video_tag("dog", array("overlay"=>"subtitles:arial_75:sample_sub_en.srt", "color"=>"#ffff0080", "background"=>"black"))
CloudinaryVideo("dog").video(overlay="subtitles:arial_75:sample_sub_en.srt", color="#ffff0080", background="black")
cloudinary.video("dog", {overlay: "subtitles:arial_75:sample_sub_en.srt", color: "#ffff0080", background: "black"})
cloudinary.url().transformation(new Transformation().overlay("subtitles:arial_75:sample_sub_en.srt").color("#ffff0080").background("black")).videoTag("dog")
$.cloudinary.video("dog", {overlay: "subtitles:arial_75:sample_sub_en.srt", color: "#ffff0080", background: "black"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Overlay("subtitles:arial_75:sample_sub_en.srt").Color("#ffff0080").Background("black")).BuildVideoTag("dog")
SRT files
SubRip Text files are text files named with the extension .srt
, and contain lines of plain text in groups of subtitles separated by a blank line. The subtitles are numbered sequentially, starting at 1 and the timecode format used is hours:minutes:seconds,milliseconds
with time units fixed to two zero-padded digits and fractions fixed to three zero-padded digits (00:00:00,000) with the comma used as a fractional separator. The file is structured as follows:
- A numeric counter identifying each sequential subtitle.
- The time that the subtitle should appear on the screen, followed by
-->
and the time it should disappear. - Subtitle text itself on one or more lines.
- A blank line containing no text, indicating the end of this subtitle.
Example of the contents of a SRT file:
1
00:00:01,000 --> 00:00:05,000
Hello World
2
00:00:06,000 --> 00:00:10,000
Cute dog shaking its head
Applying 3D LUTs to video
3D lookup tables (3D LUTs) are used to map one color space to another. They can be used to adjust colors, contrast, and/or saturation, so that you can correct contrast of flat footage, fix a camera’s inability to see a particular color shade, or give a final finished look or a particular style to your video.
After uploading a .3dl
file to your account as a raw
file, you can apply it to any video using the lut:
property of the overlay
parameter ( l_lut:
in URLs), followed by the LUT public ID (including the .3dl
extension).
Below you can see the dog.mp4
video file in it's original color, compared to the video with different LUT files applied.
cl_video_tag("dog")
cl_video_tag("dog")
CloudinaryVideo("dog").video()
cloudinary.video("dog")
cloudinary.url().videoTag("dog")
$.cloudinary.video("dog")
cloudinary.Api.UrlVideoUp.BuildVideoTag("dog")
cl_video_tag("dog", :overlay=>"lut:iwltbap_aspen.3dl")
cl_video_tag("dog", array("overlay"=>"lut:iwltbap_aspen.3dl"))
CloudinaryVideo("dog").video(overlay="lut:iwltbap_aspen.3dl")
cloudinary.video("dog", {overlay: "lut:iwltbap_aspen.3dl"})
cloudinary.url().transformation(new Transformation().overlay("lut:iwltbap_aspen.3dl")).videoTag("dog")
$.cloudinary.video("dog", {overlay: "lut:iwltbap_aspen.3dl"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Overlay("lut:iwltbap_aspen.3dl")).BuildVideoTag("dog")
cl_video_tag("dog", :overlay=>"lut:iwltbap_sedona.3dl")
cl_video_tag("dog", array("overlay"=>"lut:iwltbap_sedona.3dl"))
CloudinaryVideo("dog").video(overlay="lut:iwltbap_sedona.3dl")
cloudinary.video("dog", {overlay: "lut:iwltbap_sedona.3dl"})
cloudinary.url().transformation(new Transformation().overlay("lut:iwltbap_sedona.3dl")).videoTag("dog")
$.cloudinary.video("dog", {overlay: "lut:iwltbap_sedona.3dl"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Overlay("lut:iwltbap_sedona.3dl")).BuildVideoTag("dog")
Adaptive bitrate streaming - HLS and MPEG-DASH
Adaptive bitrate streaming is a video delivery technique that adjusts the quality of a video stream in real time according to detected bandwidth and CPU capacity. This enables videos to start quicker, with fewer buffering interruptions, and at the best possible quality for the current device and network connection, to maximize user experience.
For example, as a video begins to download, the client can play the first few seconds of the movie at a low quality to get started quickly. When the user’s video buffer is full and assuming CPU utilization is low, the client player may switch to a higher quality stream to enhance the viewing experience. If the buffer later drops below certain levels, or CPU utilization jumps above certain thresholds, the client may switch to a lower quality stream.
At the start of the streaming session, the client software downloads a master playlist file
containing the metadata for the various sub-streams that are available. The client software then decides what to download from the available media files, based on predefined factors such as device type, resolution, current bandwidth, size, etc.
To deliver videos using adaptive streaming, you need multiple copies of your video prepared at different resolutions, qualities, and data rates. These are called video representations
(also sometimes known as variants
). You also need index and streaming files for each representation. The master file references the available representations, provides information required for the client to choose between them, and includes other required metadata, depending on the delivery protocol.
Cloudinary can automatically generate and deliver all of these files from a single original video, transcoded to either or both of the following protocols:
- HTTP Live Streaming (HLS)
- Dynamic Adaptive Streaming over HTTP (MPEG-DASH)
To deliver videos using HLS or MPEG-DASH Adaptive Streaming:
- Select a predefined streaming profile
- Upload your video with an eager transformation including the selected streaming profile and format
- Deliver the video
Note: If you have a special use-case that does not enable you to use the built-in streaming profiles to automatically generate the master playlist file and all the relevant representations, you can still have Cloudinary create the index and streaming files for each streaming transformation (representation) that you define, and then you can create the master playlist file manually. For details, see Manually creating representations and master files
Step 1. Select a streaming profile
Cloudinary provides a collection of predefined streaming profiles
, where each profile defines a set of representations according to suggested best practices.
For example, the 4k
profile creates 8 different representations in 16*9 aspect ratio, from extremely high quality to audio only, while the sd
profile creates only 3 representations, all in 4:3 aspect ratio. Other commonly used profiles include the hd
and full_hd
profiles.
To view the full list of available predefined streaming profiles and the settings for each representation, see Predefined streaming profiles.
Step 2. Upload your video with an eager transformation including the streaming profile and format
A single streaming profile is comprised of many derived files, so it can take a while for Cloudinary to generate them all. Therefore, when you upload your video (or later, explicitly), you should include eager, asynchronous transformations with the required streaming profile and video format.
You can even eagerly prepare your videos for streaming in both formats and you can include other video transformations as well. However, make sure the streaming_profile is provided as a separate component of chained transformations.
For example, this upload command encodes the big_buck_bunny.mp4
video to HLS format using the full_hd
streaming profile:
Cloudinary::Uploader.upload("big_buck_bunny.mp4", :resource_type => :video, :eager => [{:streaming_profile => "full_hd", :format => "m3u8"}], :eager_async => true, :eager_notification_url => "http://mysite/notify_endpoint", :public_id => "bb_bunny")
\Cloudinary\Uploader::upload("big_buck_bunny.mp4", array( "resource_type" => "video", "eager" => array("streaming_profile" => "full_hd", "format" => "m3u8"), "eager_async" => true, "eager_notification_url" => "http://mysite/notify_endpoint" "public_id" => "bb_bunny" );
cloudinary.uploader.upload("big_buck_bunny.mp4", resource_type = "video", eager = [ {"streaming_profile": "full_hd", "format": "m3u8"}] eager_async = true, eager_notification_url = "http://mysite/notify_endpoint") public_id = "bb_bunny")
cloudinary.uploader.upload("big_buck_bunny.mp4", function(result) {console.log(result); }, { resource_type: "video", eager: [ { streaming_profile: "full_hd", format: "m3u8" }], eager_async: true, eager_notification_url: "http://mysite/notify_endpoint", public_id: "bb_bunny"});
cloudinary.uploader().upload("big_buck_bunny.mp4", ObjectUtils.asMap("resource_type", "video", "eager", Arrays.asList( new Transformation().streamingProfile("full_hd").format("m3u8"), "eager_async", true, "eager_notification_url", "http://mysite/notify_endpoint" "public_id", "bb_bunny"));
The eager transformation response includes the delivery URLs for each requested encoding.
See more adaptive streaming upload examples.
Step 3. Deliver the video
After the eager transformation is complete, deliver your video using the .m3u8
(HLS) or .mpd
(MPEG-DASH) file format (extension) and include the streaming_profile
(sp_<profilename>
) and other transformations exactly matching those you provided in your eager transformation (as per the URL that was returned in the upload response).
For example, the delivery code for the video that was uploaded in Step 2 above is:
cl_video_tag("bb_bunny.m3u8", :streaming_profile=>"hd")
cl_video_tag("bb_bunny.m3u8", array("streaming_profile"=>"hd"))
CloudinaryVideo("bb_bunny.m3u8").video(streaming_profile="hd")
cloudinary.video("bb_bunny.m3u8", {streaming_profile: "hd"})
cloudinary.url().transformation(new Transformation().streamingProfile("hd")).videoTag("bb_bunny.m3u8")
$.cloudinary.video("bb_bunny.m3u8", {streaming_profile: "hd"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().StreamingProfile("hd")).BuildVideoTag("bb_bunny.m3u8")
Important: Not all browsers and mobile devices natively support MPEG-DASH and HLS. For example, DASH is natively supported in Chrome (including Android 4.0+), Internet Explorer 11 on Windows 8.1, and many SmartTVs. HLS is supported on all Apple devices, Safari, and the latest versions of the Android browser and Chrome.
To ensure that your encoded video can stream in all environments, make sure to embed relevant client players in your application. (There are a number of open source and closed source solutions available.)
Delivering HLS version 3
By default, HLS transcoding uses HLS v4 If you need to deliver HLS v3, add the hlsv3
flag parameter (fl_hlsv3
in URLs) when setting the video file format (extension) to .m3u8
. Cloudinary will then automatically create the fragmented video files (all with a .ts extension), each with a duration of 10 seconds, as well as the required .m3u8
index files for each of the fragmented files.
Note: Supporting HLS v3 requires a private CDN configuration. CDN distributions are available for Cloudinary's Advanced plan and above, and require a small setup on Cloudinary's side. For details, submit a support request.
Notes and guidelines for adaptive streaming
For small videos, where all of the derived files combined are less than 60 MB, you can deliver the transformation URL on-the-fly (instead of as an eager transformation), but this should be used only for demo or debugging purposes. In a production environment, we recommend to always eagerly transform your video representations.
The adaptive streaming files for small videos (where all of the derived files combined are less than 60 MB) are derived synchronously if you do not set the asynch parameter. Larger videos are prepared asynchronously. Notification (webhook) of completion is sent to the
eager_notification_url
parameter.By default, video sampling is per 2 seconds. You can define a custom sampling rate by specifying it as a chained transformation prior to the streaming profile. For example (in Ruby):
Cloudinary::Uploader.upload("big_buck_bunny.mp4", :resource_type => :video, :eager => [{:format => "mpd", :transformation => [{:video_sampling => 3}, {:streaming_profile => "hd"}] }] :public_id => "bb_bunny")
All the generated files are considered derived files of the original video. For example, when performing invalidate on a video, the corresponding derived adaptive streaming files are also invalidated.
If the profile you select includes any representations larger resolution than the original, those representations will not be created. However, at minimum, one representation will be created.
If the aspect ratio of the original video does not match the aspect ratio of the selected streaming profile, then the
c_limit
cropping transformation is applied to make the video fit the required size. If you don’t want to use this cropping method, use a base transformation to crop the video to the relevant aspect ratio.If you prepare streaming for a lower-level profile, and then in the future, you want to prepare the same video with a higher-level profile, the new high-quality representations will be created, while any already existing representations that match will be reused.
Examples
Step 2 above includes a simple example for uploading a video and using a streaming profile to prepare the required files for delivering the video in adaptive streaming format. This section provides some additional examples.
Request several encodings and formats at once
Cloudinary::Uploader.upload("big_buck_bunny.mp4", :resource_type => :video, :eager => [ {:streaming_profile => "full_hd", :format => "m3u8"}, {:streaming_profile => "sd", :format => "m3u8"}, {:streaming_profile => "hd", :format => "mpd"}], :eager_async => true :eager_notification_url => "http://mysite/notify_endpoint", :public_id => "bb_bunny")
\Cloudinary\Uploader::upload("big_buck_bunny.mp4", array( "resource_type" => "video", "eager" => array( array("streaming_profile" => "full_hd", "format" => "m3u8"), array("streaming_profile" => "sd", "format" => "m3u8"), array("streaming_profile" => "hd", "format" => "mpd")), "eager_async" => true, "eager_notification_url" => "http://mysite/notify_endpoint", "public_id => "bb_bunny" ));
cloudinary.uploader.upload("big_buck_bunny.mp4", resource_type = "video", eager = [ {"streaming_profile": "full_hd", "format": "m3u8"}, {"streaming_profile": "sd", "format": "m3u8"}, {"streaming_profile": "hd", "format": "mpd"}], eager_async = true, eager_notification_url = "http://mysite/notify_endpoint", public_id = "bb_bunny")
cloudinary.v2.uploader.upload("big_buck_bunny.mp4", { resource_type: "video", eager: [ {streaming_profile: "full_hd", format: "m3u8"}, {streaming_profile: "sd", format: "m3u8"}, {streaming_profile: "hd", format: "mpd"} ], eager_async: true, eager_notification_url: "http://mysite/notify_endpoint", public_id = bb_bunny" }, function(result) {console.log(result); });
cloudinary.uploader().upload("big_buck_bunny.mp4", ObjectUtils.asMap("resource_type", "video", "eager", Arrays.asList( new Transformation().streamingProfile("full_hd").format("m3u8"), new Transformation().streamingProfile("sd").format("m3u8"), new Transformation().streamingProfile("hd").format("mpd")), "eager_async", true, "eager_notification_url", "http://mysite/notify_endpoint, "public_id", "bb_bunny"));
Use chained transformations with a streaming profile
Cloudinary::Uploader.upload("big_buck_bunny.mp4", :resource_type => :video, :eager => [{:format => "m3u8", :transformation => [ {:crop => "crop", :aspect_ratio => "16:9"}, {:streaming_profile => "hd"}, {:overlay => "logowatermark", :width => 100} ] }], :eager_async => true, :eager_notification_url => "http://mysite/notify_endpoint", :public_id => "bb_bunny")
\Cloudinary\Uploader::upload("big_buck_bunny.mp4", array( "resource_type" => "video", "eager" => array( "format" => "m3u8", "transformation" => array( array("crop" => "crop", "aspect_ratio" => "16:9"), array("streaming_profile" => "hd"), array("overlay" => "logowatermark", "width" => 100))), "eager_async" => true, "eager_notification_url" => "http://mysite/notify_endpoint", "public_id => "bb_bunny" ));
cloudinary.uploader.upload("big_buck_bunny.mp4", resource_type = "video", eager = [ {"format": "m3u8", "transformation": [ {"crop":"crop", aspect_ratio:"16:9"} {"streaming_profile": "hd"}, {"overlay": "logowatermark", ":"width": 100}] }], eager_async = true, eager_notification_url = "http://mysite/notify_endpoint", public_id = "bb_bunny")
cloudinary.v2.uploader.upload("big_buck_bunny.mp4", { resource_type: "video", eager: [ {format: "m3u8", transformation: [ {crop: "crop", aspect_ratio: "16:9"}, {streaming_profile: "hd"}, {:overlay: "logowatermark", width: 100} ]}, ], eager_async: true, eager_notification_url: "http://mysite/notify_endpoint", public_id = bb_bunny" }, function(result) {console.log(result); });
cloudinary.uploader().upload("big_buck_bunny.mp4", ObjectUtils.asMap("resource_type", "video", "eager", Arrays.asList( new Transformation() .format("m3u8").chain() .crop("crop").aspectRatio("16:9").chain() .streamingProfile("hd").chain() .overlay("logowatermark").width(100) ) "eager_async", true, "eager_notification_url", "http://mysite/notify_endpoint, "public_id", "bb_bunny"));
Encode an already uploaded video using Explicit
Cloudinary::Uploader.explicit("bb_bunny", :resource_type => :video, :type => "upload", :eager => [ {:streaming_profile => "hd", :format => "m3u8"}])
\Cloudinary\Uploader::explicit("bb_bunny", array( "resource_type" => "video", "type" => "upload" "eager" => array( array("streaming_profile" => "hd", "format" => "m3u8"), )));
cloudinary.uploader.explicit("bb_bunny", resource_type = "video", type = "upload", eager = [ {"streaming_profile": "hd", "format": "m3u8"}, ])
cloudinary.v2.uploader.explicit("bb_bunny", { resource_type: "video", type: "upload", eager: [ { streaming_profile: "hd", format: "m3u8" }, ] } function(result) {console.log(result); } );
cloudinary.uploader().explicit("bb_bunny", ObjectUtils.asMap( "resource_type", "video", "type", "upload", "eager", Arrays.asList( new Transformation().streaming_profile("hd").format("m3u8"), )));
Eager response example
{
"public_id": "bb_bunny",
"version": 1466426766,
"signature": "245679585758d518703ab50142b1c88685e17777",
"width": 640,
"height": 360,
"format": "mp4",
"resource_type": "video",
"created_at": "2016-06-20T12:46:06Z",
"bytes": 5510872,
"type": "upload",
"url": "http://res.cloudinary.com/demo/video/upload/v1466426766/bb_bunny.mp4",
"secure_url": "https://res.cloudinary.com/demo/video/upload/v1466426766/bb_bunny.mp4",
"eager": [
{
"transformation": "sp_hd/m3u8",
"url": "http://res.cloudinary.com/demo/video/upload/sp_hd/v1466426766/bb_bunny.m3u8",
"secure_url": "https://res.cloudinary.com/demo/video/upload/sp_hd/v1466426766/bb_bunny.m3u8"
}
]
}
Predefined streaming profiles
The following tables summarize the possible representations, and the actual representations that are included for each predefined streaming profile.
Possible representations
ID | Width | Height | Video Codec/Profile (video_codec) |
Video Bitrate (bit_rate) |
---|---|---|---|---|
0 | 320 | 240 | (Audio only) | 192k |
1 | 480 | 270 (360 in 4:3 ratio) |
h264:baseline:3.0 | 800k |
2 | 640 | 360 (480 in 4:3 ratio) |
h264:baseline:3.0 | 2m |
3 | 960 | 540 | h264:main:3.1 | 3500k |
4 | 1280 | 720 | h264:main:3.1 | 5500k |
5 | 1920 | 1080 | h264:high:4.0 | 8500k |
6 | 2560 | 1440 | h264:high:4.0 | 16m |
7 | 3840 | 2160 | h264:high:4.0 | 35m |
Representations generated for each profile
Profile Name | Aspect Ratio | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 |
---|---|---|---|---|---|---|---|---|---|
4k | 16:9 | + | + | + | + | + | + | + | + |
full_hd | 16:9 | + | + | + | + | + | + | ||
hd | 16:9 | + | + | + | + | + | |||
sd | 4:3 | + | + | + | |||||
full_hd_wifi | 16:9 | + | + | + | |||||
full_hd_lean | 16:9 | + | + | + | |||||
hd_lean | 16:9 | + | + | + |
Derived adaptive streaming files
When the encoding process is complete for all representations, the derived files will include:
HLS
- A fragmented video streaming file (.ts) 1 for each representation
- An index file (.m3u8) of references to the fragments for each representation
- A single master playlist file (.m3u8) containing references to the representation files above and other required metadata
DASH
- A fragmented video streaming file (.mp4dv) for each representation
- An audio stream file (.mp4da) for each representation
- An index file (.mpd) containing references to the fragments of each streaming file as well as a master playlist containing references to the representation files above and other required metadata
1 If working with HLS v.3, a separate video streaming file (.ts) is derived for each fragment of each representation. To work with HLS v.3, you need a private CDN configuration and you need to add a special hlsv3 flag to your video transformations.
Manually creating representations and master files
If you have a special use-case that does not enable you to use the built-in streaming profiles to automatically generate master playlist file and all the relevant representations, you can still have Cloudinary create the index and streaming files for each streaming transformation you define, and then you can create the master playlist file manually.
Step 1. Upload your video with eager transformations for each desired representation
When you upload your video, provide eager transformations for each representation (resolution, quality, data rate) that you want to create. Also make sure to define the format
for each transformation as .m3u8
(HLS) or .mpd
(DASH) in a separate component of the chain.
When you define the format of your transformation as .m3u8
or .mpd
, Cloudinary automatically generates the following derived files:
- A fragmented video streaming file (
.ts
1 or.mp4dv
) - An index file (
.m3u8
or.mpd
) of references to the fragments - For DASH only: an audio stream file (
.mp4da
)
For example:
Cloudinary::Uploader.upload("big_buck_bunny.mp4", :resource_type => :video, :eager => [{:format => "m3u8", :transformation => [ {:width => 960, :height => 540, :crop => :limit, :video_codec => "h264:main:3.1", :bit_rate =>"3500k"}, {:overlay => "watermark", :width => 100} ] }], :eager_async => true, :eager_notification_url => "http://mysite/notify_endpoint") :public_id => "bb_bunny"
\Cloudinary\Uploader::upload("big_buck_bunny.mp4", array( "resource_type" => "video", "eager" => array( array("width" => 960, "height" => 540, "crop" => "limit", "video_codec" => "h264:main:3.1", "bit_rate" =>"3500k") ), "eager_async" => true, "eager_notification_url" => "http://mysite/notify_endpoint" ״public_id => "bb_bunny" ));
cloudinary.uploader.upload("big_buck_bunny.mp4", resource_type = "video", eager = [ {"width": 960, "height": 540, "crop": "limit", "video_codec": "h264:main:3.1", "bit_rate": "3500k"} ], eager_async = true, eager_notification_url = "http://mysite/notify_endpoint" public_id = "bb_bunny" )
cloudinary.v2.uploader.upload("big_buck_bunny.mp4", { resource_type: "video", eager: [ { width: 960, height: 540, crop: "limit", video_codec: "h264:main:3.1", bit_rate: "3500k" } ], eager_async: true, eager_notification_url: "http://mysite/notify_endpoint" public_id: "bb_bunny" } function(result) {console.log(result); });
cloudinary.uploader().upload("big_buck_bunny.mp4", ObjectUtils.asMap("resource_type", "video", "eager", Arrays.asList( new Transformation().width(960).height(540).crop("limit").videoCodec("h264:main:3.1").bitRate("3500k")), "eager_async", true, "eager_notification_url", "http://mysite/notify_endpoint" "public_id", "bb_bunny"));
Note: It is possible to create adaptive streaming transformations on the fly, but recommended to you create all the required transformations eagerly when uploading the original video file or explicitly, so that there is no delay when first accessed by your users.
1If working with HLS v.3, a separate video streaming file (.ts) is derived for each fragment of each representation. To work with HLS v.3, you need a private CDN configuration and you need to add a special hlsv3 flag to your video transformations.
Step 2. Manually create the master playlist file
If you are not using streaming profiles, you need to create your master playlist file manually.
HLS Master Playlist (m3u8)
Each item in the m3u8
master index file points to a Cloudinary dynamic manipulation URL, which represents the pair of auto-generated m3u8
index and ts
video files that were created in the preceding step.
For example, the following m3u8 master playlist file contains information on 2 versions of the dog.mp4
video, one high resolution suitable for iPhone 4s and the other lower resolution suitable for iPhone 1s:
#EXTM3U
#EXT-X-STREAM-INF:BANDWIDTH=5000000,RESOLUTION=480x320,NAME="Nice Dog - iPhone 4" http://res.cloudinary.com/demo/video/upload/c_lpad,w_960,h_640,vs_2,q_70,br_5m,vc_h264:main:3.1/dog.m3u8
#EXT-X-STREAM-INF:BANDWIDTH=1500000,RESOLUTION=480x320,NAME="Nice Dog - iPhone 1" http://res.cloudinary.com/demo/video/upload/c_lpad,w_480,h_320,vs_2,q_70,br_1400k,vc_h264:baseline:3.0/dog.m3u8
MPEG-DASH Master Playlist
The .mpd
master file is an XML document. For details on creating the .mpd
master file, see: The Structure of an MPEG-DASH MPD.
Audio manipulation and streaming
Cloudinary's audio support includes uploading any audio file to your account, converting the audio file format, extracting audio from videos and manipulating audio files on delivery. Audio files can be uploaded to Cloudinary as a "video" resource (see the Upload documentation for more information). For example, uploading a local audio file named audio_sample.mp3:
Cloudinary::Uploader.upload("audio_sample.mp3", :resource_type => :video)
\Cloudinary\Uploader::upload("audio_sample.mp3", array("resource_type" => "video"));
cloudinary.uploader.upload("audio_sample.mp3", resource_type = "video")
cloudinary.uploader.upload("audio_sample.mp3", { resource_type: "video" } function(result) {console.log(result); });
cloudinary.uploader().upload("audio_sample.mp3", ObjectUtils.asMap("resource_type", "video"));
Video files can be converted to audio files by setting the file format (or extension) to one of the supported audio formats: MP3, OGG or WAV (see Transcoding videos for more information). For example, to convert the MP4 video file named dog
to an MP3 audio file:
cl_video_tag("dog.mp3")
cl_video_tag("dog.mp3")
CloudinaryVideo("dog.mp3").video()
cloudinary.video("dog.mp3")
cloudinary.url().videoTag("dog.mp3")
$.cloudinary.video("dog.mp3")
cloudinary.Api.UrlVideoUp.BuildVideoTag("dog.mp3")
dog
to an MP3 audio file:
cl_video_tag("dog.mp3", :start_offset=>"0", :duration=>"3")
cl_video_tag("dog.mp3", array("start_offset"=>"0", "duration"=>"3"))
CloudinaryVideo("dog.mp3").video(start_offset="0", duration="3")
cloudinary.video("dog.mp3", {start_offset: "0", duration: "3"})
cloudinary.url().transformation(new Transformation().startOffset("0").duration("3")).videoTag("dog.mp3")
$.cloudinary.video("dog.mp3", {start_offset: "0", duration: "3"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().StartOffset("0").Duration("3")).BuildVideoTag("dog.mp3")
Creating animated GIF and animated WebP
Cloudinary supports 2 formats for delivering your videos as animated images: GIF
and WebP
. There are a quite a few advantages to using the WebP format including:
- WebP supports 24-bit RGB color with an 8-bit alpha channel, compared to GIF's 8-bit color and 1-bit alpha.
- WebP supports both lossy and lossless compression (a single animation can combine lossy and lossless frames), well-suited to animated images created from real-world videos. GIF only supports lossless compression.
- WebP requires fewer bytes than GIF, ranging from 64% smaller for Animated GIF converted to lossy WebP, to 19% smaller for lossless WebP.
The disadvantage is that whereas animated GIF are universally supported, WebP is only supported by the Chrome and Opera web browsers.
This section is divided into the following subsections:
Delivering animated GIFs
Delivering an animated GIF that is generated from an uploaded video is as simple as changing the file extension of the URL to .gif
. Converting a video to animated GIF is normally done while resizing and cropping to match your site (usually scaling down). By default, the resulting animated GIF is generated from the whole video (up to 400 frames, at up to 10 frames per second) and is the same duration as the original video no matter how many frames are sampled. The resulting animated GIFs can also be further manipulated like any other image uploaded to Cloudinary.
To control how many frames are taken from the original video and the speed that they are displayed, use the video_sampling
and delay
parameters.
The video_sampling
parameter (vs
in URLs) determines how many frames to sample from the original video and can take one of the following values:
Integer - The total number of frames to sample from the original video. The frames are spread out over the length of the video, e.g.
25
samples one frame every 4%.String - A float with an s appended representing the number of seconds between each frame to sample from the original video. e.g.
2.3s
samples one frame every 2.3 seconds.
The delay
parameter (dl
in URLs) is used to set the time delay between the individual frames of the animated GIF, in milliseconds.
Examples of generating an animated GIF from the uploaded mp4 video named dog
:
Using a sample of 20 frames from the original video and setting a delay of 200 milliseconds between the frames of the resulting animated GIF, which is scaled down to a width of 300 pixels:
Ruby:cl_image_tag("dog.gif", :video_sampling=>"25", :delay=>"200", :width=>300, :resource_type=>"video")
PHP:cl_image_tag("dog.gif", array("video_sampling"=>"25", "delay"=>"200", "width"=>300, "resource_type"=>"video"))
Python:CloudinaryVideo("dog.gif").image(video_sampling="25", delay="200", width=300)
Node.js:cloudinary.image("dog.gif", {video_sampling: "25", delay: "200", width: 300, resource_type: "video"})
Java:cloudinary.url().transformation(new Transformation().videoSampling("25").delay("200").width(300)).resourceType("video").imageTag("dog.gif")
jQuery:$.cloudinary.image("dog.gif", {video_sampling: "25", delay: "200", width: 300, resource_type: "video"})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().VideoSampling("25").Delay("200").Width(300)).BuildImageTag("dog.gif")
Sampling one frame every 1.1 seconds from the original video and setting a delay of 100 milliseconds between the frames of the resulting animated GIF, which is scaled down to a width of 200 pixels:
Ruby:cl_image_tag("dog.gif", :video_sampling=>"1.1s", :delay=>"100", :width=>200, :resource_type=>"video")
PHP:cl_image_tag("dog.gif", array("video_sampling"=>"1.1s", "delay"=>"100", "width"=>200, "resource_type"=>"video"))
Python:CloudinaryVideo("dog.gif").image(video_sampling="1.1s", delay="100", width=200)
Node.js:cloudinary.image("dog.gif", {video_sampling: "1.1s", delay: "100", width: 200, resource_type: "video"})
Java:cloudinary.url().transformation(new Transformation().videoSampling("1.1s").delay("100").width(200)).resourceType("video").imageTag("dog.gif")
jQuery:$.cloudinary.image("dog.gif", {video_sampling: "1.1s", delay: "100", width: 200, resource_type: "video"})
.Net:cloudinary.Api.UrlVideoUp.Transform(new Transformation().VideoSampling("1.1s").Delay("100").Width(200)).BuildImageTag("dog.gif")
Delivering animated WebP files
Delivering an animated WebP that is generated from an uploaded video is similar to generating an animated GIF as described above, except that you also need to enable the awebp
and animated
flags (fl_awebp
and fl_animated
in URLs).
For example, generating an animated WebP from the uploaded mp4 video named dog
, using a sample of 10 frames from the original video and setting a delay of 100 milliseconds between the frames of the resulting animated WebP, which is also scaled down to a width of 250 pixels:
cl_video_tag("dog.webp", :video_sampling=>"10", :delay=>"100", :width=>250, :flags=>["awebp", "animated"])
cl_video_tag("dog.webp", array("video_sampling"=>"10", "delay"=>"100", "width"=>250, "flags"=>array("awebp", "animated")))
CloudinaryVideo("dog.webp").video(video_sampling="10", delay="100", width=250, flags=["awebp", "animated"])
cloudinary.video("dog.webp", {video_sampling: "10", delay: "100", width: 250, flags: ["awebp", "animated"]})
cloudinary.url().transformation(new Transformation().videoSampling("10").delay("100").width(250).flags("awebp", "animated")).videoTag("dog.webp")
$.cloudinary.video("dog.webp", {video_sampling: "10", delay: "100", width: 250, flags: ["awebp", "animated"]})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().VideoSampling("10").Delay("100").Width(250).Flags("awebp", "animated")).BuildVideoTag("dog.webp")

Note that you can also create animated images from videos together with the auto
format and the animated
flag parameters (f_auto
and fl_animated
in URLs). Adding these parameters will deliver an animated WebP to browsers that support the format, and fall back to delivering an animated GIF to browsers that don't support the format. See the blog post on animated WebP for more information.
For example, to deliver an animated WebP of the mp4 video named dog
to supported browsers, but fall back to animated GIF for unsupported browsers:
cl_image_tag("dog.gif", :width=>250, :flags=>"animated", :fetch_format=>:auto, :resource_type=>"video")
cl_image_tag("dog.gif", array("width"=>250, "flags"=>"animated", "fetch_format"=>"auto", "resource_type"=>"video"))
CloudinaryVideo("dog.gif").image(width=250, flags="animated", fetch_format="auto")
cloudinary.image("dog.gif", {width: 250, flags: "animated", fetch_format: "auto", resource_type: "video"})
cloudinary.url().transformation(new Transformation().width(250).flags("animated").fetchFormat("auto")).resourceType("video").imageTag("dog.gif")
$.cloudinary.image("dog.gif", {width: 250, flags: "animated", fetch_format: "auto", resource_type: "video"})
cloudinary.Api.UrlVideoUp.Transform(new Transformation().Width(250).Flags("animated").FetchFormat("auto")).BuildImageTag("dog.gif")

Video transformations reference
Library Parameter | URL Parameter | Value | Description |
---|---|---|---|
Resizing and cropping videos | |||
width | w | Integer or float | The required width of a transformed video. |
80 | An integer value resizing width to 80 pixels. | ||
0.2 | A float value resizing video to 20% of its original size. | ||
height | h | Integer or float | The required height of a transformed video. |
40 | An integer value resizing height to 40 pixels. | ||
0.3 | A float value resizing video to 30% of its original size. | ||
crop | c | String | A crop mode that determines how to transform the video for fitting into the desired width & height dimensions. |
scale | Change the size of the video to match the given width and/or height. All original video parts will be visible and are stretched if necessary to fill the new dimensions, This is the default cropping mode. | ||
fill | Create a video with the exact given width and height while retaining original proportions. Uses only a portion of the original video that fills the given dimensions. | ||
fit | Change video size to fit in the given width and height while retaining original proportions. All original video parts are visible and the resulting image will fit in the given dimensions, maintaining aspect ratio and scaling up or down as needed. | ||
limit | Used for creating a video that does not exceed the given width or height while retaining original proportions. All original video parts are visible and scaled down if needed. | ||
pad | Scale the video up or down as needed to fit in the given width and height while retaining original proportions. Padding will be added if the original video proportions do not match the required ones. | ||
lpad | Same as the 'pad' mode but doesn't scale the video up if your requested dimensions are larger than the original video's. | ||
crop | Used to extract a given width and height out of the original video. The original proportions are retained and so is the size of the graphics. Used together with x and y coordinates for specifying the section to crop (or with the gravity parameter). | ||
aspect_ratio | ar | String or float | Resizes the video to a new aspect ratio. |
4:3 | A string value in the form a:b, where a is the width and b is the height. The example value resizes the aspect ratio to 4/3. | ||
2.5 | A decimal value representing the width divided by the height. The example value resizes the aspect ratio to 5/2. | ||
gravity | g | String | Decides which part of the video to keep while 'crop', 'pad' and 'fill' crop modes are used. For overlays, this decides where to place the overlay. |
north_west | North west corner (top left). | ||
north | North center part (top center). | ||
north_east | North east corner (top right). | ||
west | Middle west part (left). | ||
center | The center of the video (default value). | ||
east | Middle east part (right). | ||
south_west | South west corner (bottom left). | ||
south | South center part (bottom center). | ||
south_east | South east corner (bottom right). | ||
background | b | String | Defines the background color to use in the case of padding. |
blue | Fill the background with a named color. | ||
rgb:9090ff | Fill the background with the color defined by a RGB hex triplet. Client libraries support a # shortcut (e.g. #9090ff). | ||
rgb:999 | Fill the background with the color defined by a 3 character RGB value. Client libraries support a # shortcut (e.g. #999). | ||
Rotating videos | |||
angle | a | Integer | Rotate a video by the given degrees. |
90 | Rotate video by 90 degrees clockwise. | ||
-20 | Rotate video by 20 degrees counterclockwise. | ||
Video Settings | |||
format or fetch_format | f (or file extension) | String | Format conversion to the given web format while normalizing and optimizing for web and mobile viewing. |
webm | Convert a video to the WebM format. | ||
mp4 | Convert a video to the MP4 format. | ||
ogv | Convert a video to the OGV format. | ||
flv | Convert a video to the FLV format. | ||
m3u8 | Create the index and fragment files necessary for HTTP Live Streaming (HLS). | ||
video_codec | vc | String | Select the video codec and control the video content of the profile used (the profile mainly controls which devices/browsers to support). |
auto | Normalize and optimize the video for web viewing with default settings.
Default settings applied for each format:
MP4Video codec: h264, profile: baseline, quality: 70, audio codec: aac, audio frequency: 22050. WebMVideo codec: vp8, quality: 70, audio codec: vorbis, audio frequency: 22050. OGVVideo codec: theora, quality: 70, audio codec: vorbis, audio frequency: 22050. FLVVideo codec: h264, profile: high, quality: 70, audio codec: aac, audio frequency: 22050. | ||
<codec>[:<profile>:[<level>]] | Specific values to apply for video codec, profile and level. Examples:
| ||
quality | q | Integer | Control the video quality. 1 is the lowest quality and 100 is the highest. Reducing quality generates videos smaller in file size. See the video_codec parameter for the default values for each format. |
50 | Generate a video using a low quality of 50. | ||
bit_rate | br | Integer or String | Advanced control of video bit rate in bits per second. |
200000 | Integer number of bits per second. | ||
500k | String supporting 'k' and 'm’ for kilobits and megabits respectively e.g., '500k' or ?1m'. The 'k' and 'm' are supported on the server-side. | ||
Video Effects | |||
effect | e | String:Integer | Apply a filter or an effect on a video. The value includes the name of the effect and an additional parameter that controls the behavior of the specific effect. |
accelerate:100 | Speeds up the video playback speed by %100. A negative value would slow down the playback speed (Range: -50 to 100 Default: 0). | ||
noise:10 | Adds 10% visual noise to the video, visible as a random flicker of "dots" or "snow" (Range: 0 to 100 Default: 0). | ||
deshake:32 | Remove small motion shifts from the video with a maximum extent of movement in the horizontal and vertical direction of 32 pixels (Range: 16, 32, 48 or 64 Default: 16). | ||
fade:2000 | Fade in to the beginning of the video over 2000 milliseconds. A negative value would fade out at the end of the video (Default: 1000). | ||
contrast:50 | Increase the contrast by 50. | ||
brightness:30 | Increase the brightness by 30. | ||
saturation:-20 | Decrease the saturation by 20. | ||
gamma:15 | Increase the gamma by 15. | ||
vignette:40 | Apply a vignette effect with a value of 40. | ||
volume:70 | Increase the volume by 70%. | ||
reverse | Play the video or audio file in reverse. | ||
Video Flags | |||
flags | f | String | Set a flag that alters the default transformation behavior. |
splice | Splice the video stipulated as an overlay on to the end of the container video instead of adding it as an overlay. | ||
layer_apply | Apply all chained transformations, until a transformation component that includes this flag, on the last added overlay instead of applying them on the containing video. | ||
no_stream | Don't stream a video that is currently being generated on the fly. Wait until the video is fully generated. | ||
truncate_ts | Truncate (trim) a video file according to the included metadata (relevant only where the metadata includes a directive to play only a section of the video). | ||
waveform | Create a waveform image (in the format specified by the file extension) from the audio or video file. | ||
Audio Settings | |||
audio_codec | ac | String | Control the audio codec or remove the audio channel |
none | Remove audio channel | ||
aac | Set audio codec to aac (mp4 or flv only) | ||
vorbis | Set audio codec to vorbis (ogv or webm only) | ||
mp3 | Set audio codec to mp3 (mp4 or flv only) | ||
audio_frequency | af | Integer | Control audio sample frequency. |
44100 | This parameter represents an integer value in Hz and can only take one of the following values: 8000, 11025, 16000, 22050, 32000, 37800, 44056, 44100, 47250, 48000, 88200, 96000, 176400 or 192000. | ||
Animated GIFs and animated WebPs | |||
video_sampling | vs | Integer or String | Relevant for conversion of video to animated GIF or WebP. If not specified, the resulting GIF or WebP samples the whole video (up to 400 frames, at up to 10 frames per second). By default the duration of the animated image is the same as the duration of the video, no matter how many frames are sampled from the original video (use the delay parameter to adjust the amount of time between frames). |
20 | Integer - The total number of frames to sample from the original video. The frames are spread out over the length of the video, e.g. 20 takes one frame every 5%. | ||
'2.3s' | String - The number of seconds between each frame to sample from the original video. e.g. 2.3s takes one frame every 2.3 seconds. | ||
delay | dl | Integer | Controls the time delay between the frames of an animated image, in milliseconds. |
20 | Sets the delay between frames of the animated image to 20 milliseconds. | ||
Adding overlays | |||
overlay | l | String | Add an overlay over the base video. You can control the dimension and position of the overlay using the width, height, x, y and gravity parameters. The string identifier is the public ID of an uploaded image you want to overlay or one of the special overlay types. |
badge | Add the overlay of an uploaded image with the public ID 'badge'. | ||
text:Roboto_30p:Hello World | Add a 'Hello World' text overlay using the Roboto font and 30px size characters. | ||
subtitles:sample.srt | Add subtitles as specified in the 'sample.srt' file to the video. | ||
subtitles:arial_20:sample.srt | Add subtitles in 20 pixel Arial font as specified in 'sample.srt'. | ||
lut:lut_name.3dl | Apply the specified 3D LUT file to the video. | ||
video:dog | Add the overlay of an uploaded video with the public ID 'dog'. | ||
Offset parameters for Trimming and Overlays | |||
start_offset | so | Float or string | Offset in seconds or percent of a video, normally used together with the end_offset and duration parameters. Used to specify one of the following:
|
2.63 | Float representing seconds | ||
'35p' | String representing a percent value | ||
'35%' | String representing a percent value (only supported in client libraries and is converted to 35p in the generated URL.). | ||
auto | Automatically selects a suitable frame from the first few seconds of the video (only relevant for generating image thumbnails). | ||
end_offset | eo | Float or string | Offset in seconds or percent of a video, normally used together with the start_offset and duration parameters. Used to specify:
|
5.33 | Float representing seconds. | ||
'75p' | String representing a percent value. | ||
'55%'' | String representing a percent value (only supported in client libraries and is converted to 55p in the generated URL). | ||
duration | du | Float or string | Offset in seconds or percent of a video, normally used together with the start_offset and end_offset parameters. Used to specify:
|
6.12 | Float representing seconds | ||
'60p' | String representing a percent value. | ||
'40%' | String representing a percent value (only supported in client libraries and is converted to 40p in the generated URL). | ||
offset | - | [float, float] or [string, string] or a range | Shortcut to set video cutting using a combination of start_offset and end_offset values. Client library only. |
[2.66, 3.21] | Float values in seconds. | ||
['35%', '70%'] | Strings representing percent values. | ||
['35p', '70p'] | Strings representing percent values. | ||
2.66..3.21 or '35p..65p' | Range of float values in seconds, or range of strings representing percent values (in frameworks supporting ranges only). | ||