Fetch remote images
Overview
Cloudinary offers an easy solution to uploading all your static and dynamic resources to the cloud by means of our secure API (either directly via HTTPS or through our client libraries) and the Cloudinary Management Console. When it comes to existing remote images, Cloudinary offers a powerful feature for automatically fetching, manipulating and delivering the images, using dynamic URLs.
Auto Upload and Fetch are two similar features for automatically fetching (retrieving) images from existing remote locations using dynamic URLs. The images are delivered through a powerful CDN network, greatly improving your users’ browsing experience, reduce load on your servers and lower hosting costs, while you also benefit from on-the-fly image manipulation and optimization, and all of this with minimum changes on your side.
The main difference between these two features is how the remote image is handled:
Fetch enables on-the-fly manipulation of existing remote images and optimized delivery via a CDN. Fetched images are cached on your Cloudinary account for performance reasons.
Auto Upload also enables on-the-fly manipulation of existing remote images and optimized delivery via a CDN, while simultaneously uploading the image to your Cloudinary account for further management, and thus benefiting from a variety of additional features (just like any other image uploaded to your Cloudinary account).
Remote image Fetch URL
The Fetch feature is a quick way to deliver images from remote URLs. The image can be manipulated and optimized on-the-fly, before being cached and delivered through fast, localized CDNs and not via local web servers. To create a Fetch URL, simply prepend the following prefix to the URL of the image:
http://res.cloudinary.com/<your Cloudinary account's cloud name>/image/fetch/
For example: Assuming the following image of Scarlett Johansson from Wikipedia is also displayed on your website:
http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg
Access the following URL to fetch the image via Cloudinary:
cl_image_tag("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg", :type=>"fetch")
cl_image_tag("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg", array("type"=>"fetch"))
CloudinaryImage("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg").image(type="fetch")
cloudinary.image("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg", {type: "fetch"})
cloudinary.url().type("fetch").imageTag("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg")
$.cloudinary.image("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg", {type: "fetch"})
cloudinary.Api.UrlImgUp.Type("fetch").BuildImageTag("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg")

Both URLs return the exact same image, only the second one is cached and delivered through fast, localized CDNs and not via the local web server.
Note: For customers with a Custom Domain Name (CNAME - available for Cloudinary's Advanced plan and above), prepend the following prefix to the URL of the image:
http://<your custom domain name>/image/fetch/
Fetch URL with on-the-fly image manipulation
You can also use the Fetch feature to apply any of Cloudinary’s image transformations to the delivered image. Simply add the transformation parameters to the URL directly after the Fetch prefix and before the URL of the image.
For example, the following Cloudinary URL delivers an image of Scarlett Johansson from Wikipedia that has been manipulated as follows: the width and height parameters are both set to 300 pixels, the image is cropped to fill in the new dimensions, retaining the original proportions and setting gravity to the detected face; the image is also made circular by setting the radius parameter to maximum, and finally, depending on the browser/device, the best image format (WebP or JPEG-XR) is automatically delivered optimized and cached via CDN:
cl_image_tag("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg", :width=>300, :height=>300, :gravity=>"face", :radius=>"max", :crop=>"fill", :fetch_format=>:auto, :type=>"fetch")
cl_image_tag("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg", array("width"=>300, "height"=>300, "gravity"=>"face", "radius"=>"max", "crop"=>"fill", "fetch_format"=>"auto", "type"=>"fetch"))
CloudinaryImage("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg").image(width=300, height=300, gravity="face", radius="max", crop="fill", fetch_format="auto", type="fetch")
cloudinary.image("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg", {width: 300, height: 300, gravity: "face", radius: "max", crop: "fill", fetch_format: "auto", type: "fetch"})
cloudinary.url().transformation(new Transformation().width(300).height(300).gravity("face").radius("max").crop("fill").fetchFormat("auto")).type("fetch").imageTag("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg")
$.cloudinary.image("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg", {width: 300, height: 300, gravity: "face", radius: "max", crop: "fill", fetch_format: "auto", type: "fetch"})
cloudinary.Api.UrlImgUp.Transform(new Transformation().Width(300).Height(300).Gravity("face").Radius("max").Crop("fill").FetchFormat("auto")).Type("fetch").BuildImageTag("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg")

Note that if the image behind the original URL changes, Cloudinary will not automatically update the fetched image embedded in your site and all its transformed versions (if you need to update fetched images, contact Cloudinary).
Creating a Fetch URL via code
Cloudinary’s SDKs make it easy to build URLs and include methods for creating generic HTML image tags. To use the Fetch feature via code, set the type
parameter of the image tag method to fetch
. For example, the following code snippet creates an image tag to the same transformed image as shown above:
cl_image_tag("http://upload.wikimedia.org/wikipedia/commons/0/0c/Scarlett_Johansson_Césars_2014.jpg" :type => :fetch, :width => 300, :height => 300, :crop => :crop, :gravity => :north_west, :radius => :max, :effect => :sharpen, :fetch_format => :auto)
Creating a Fetch URL containing special characters
URLs containing special characters (particularly the ? character) need to be modified (or escaped) for use with the Fetch feature. This is relevant for any special characters that are not allowed “as is” in a valid URL path, as well as other special unicode characters. These URLs should be escaped using % based URL encoding to ensure the URL is valid. Either escape the individual special characters themselves within the URL (for example, replacing ?
with %3F
) or escape the whole URL.
Note that the methods within Cloudinary’s client libraries (e.g. the Ruby on Rails cl_image_tag
method) automatically perform smart escaping, leaving the URL as simple as possible while escaping certain special characters. Manually escaping URLs as explained here is only relevant when simply adding the Fetch prefix to existing URLs.
For example, the original URL:
https://encrypted-tbn2.gstatic.com/images?q=tbn:ANd9GcSVmwds9qedbHZ__uc1E40a-s70KtNiiLVKiaKymGszYdIDtzJfzw
Fully escaped becomes:
cl_image_tag("https://encrypted-tbn2.gstatic.com/images?q=tbn:ANd9GcSVmwds9qedbHZ__uc1E40a-s70KtNiiLVKiaKymGszYdIDtzJfzw", :type=>"fetch")
cl_image_tag("https://encrypted-tbn2.gstatic.com/images?q=tbn:ANd9GcSVmwds9qedbHZ__uc1E40a-s70KtNiiLVKiaKymGszYdIDtzJfzw", array("type"=>"fetch"))
CloudinaryImage("https://encrypted-tbn2.gstatic.com/images?q=tbn:ANd9GcSVmwds9qedbHZ__uc1E40a-s70KtNiiLVKiaKymGszYdIDtzJfzw").image(type="fetch")
cloudinary.image("https://encrypted-tbn2.gstatic.com/images?q=tbn:ANd9GcSVmwds9qedbHZ__uc1E40a-s70KtNiiLVKiaKymGszYdIDtzJfzw", {type: "fetch"})
cloudinary.url().type("fetch").imageTag("https://encrypted-tbn2.gstatic.com/images?q=tbn:ANd9GcSVmwds9qedbHZ__uc1E40a-s70KtNiiLVKiaKymGszYdIDtzJfzw")
$.cloudinary.image("https://encrypted-tbn2.gstatic.com/images?q=tbn:ANd9GcSVmwds9qedbHZ__uc1E40a-s70KtNiiLVKiaKymGszYdIDtzJfzw", {type: "fetch"})
cloudinary.Api.UrlImgUp.Type("fetch").BuildImageTag("https://encrypted-tbn2.gstatic.com/images?q=tbn:ANd9GcSVmwds9qedbHZ__uc1E40a-s70KtNiiLVKiaKymGszYdIDtzJfzw")
Fetch URL with minimal escaping:
Restricting the allowed Fetch domains
You can restrict the list of allowed domains that can be used with the Fetch feature in the Allowed fetch domains section on the Security tab of the Settings page in Cloudinary's Management Console.
Auto upload remote images
The Auto Upload feature combines the advantages of dynamic image fetching from existing online locations, with the advantages of managing images directly in the cloud (listing, editing, browsing, tagging, transforming, moderating, dynamically manipulating, viewing reports, and more) via the Cloudinary Management Console. The Auto Upload feature enables on-the-fly manipulation of existing remote images and optimized delivery via a CDN, while automatically uploading the image to your Cloudinary account for further management and manipulation.
The Auto Upload feature is implemented by mapping a base remote URL to a specified folder in your Cloudinary account. Then, whenever accessing a Cloudinary delivery URL containing the folder prefix, any resources are automatically retrieved from the mapped URL if they are not already uploaded to the folder, and are available for further manipulation, management and CDN delivery like any other image uploaded to Cloudinary.
Mapping a remote URL prefix also allows your Cloudinary delivery URLs to be shorter and more SEO friendly, gives you fine-grained control on which remote domains are allowed for automatic uploads, enables lazy migration of all your existing images to the cloud as well as other powerful origin pulling options.
The Auto Upload URL takes the following structure:
http://res.cloudinary.com/<your Cloudinary account's cloud name>/image/upload/<mapped upload folder prefix>/<partial path of remote image>
For example: Creating a folder called remote_media
and then mapping it to the URL prefix http://upload.wikimedia.org/wikipedia/
allows you to generate a Cloudinary delivery URL that substitutes the remote_media folder prefix for the URL prefix. When the Cloudinary delivery URL is accessed for the first time, Cloudinary automatically retrieves the remote image from http://upload.wikimedia.org/wikipedia/ and stores it in your Cloudinary account.
To retrieve the following image from Wikimedia, and automatically upload it to your Cloudinary account:
http://upload.wikimedia.org/wikipedia/commons/2/29/Marcelo_Facini.jpg
Access the following Cloudinary URL:
cl_image_tag("remote_media/commons/2/29/Marcelo_Facini.jpg")
cl_image_tag("remote_media/commons/2/29/Marcelo_Facini.jpg")
CloudinaryImage("remote_media/commons/2/29/Marcelo_Facini.jpg").image()
cloudinary.image("remote_media/commons/2/29/Marcelo_Facini.jpg")
cloudinary.url().imageTag("remote_media/commons/2/29/Marcelo_Facini.jpg")
$.cloudinary.image("remote_media/commons/2/29/Marcelo_Facini.jpg")
cloudinary.Api.UrlImgUp.BuildImageTag("remote_media/commons/2/29/Marcelo_Facini.jpg")
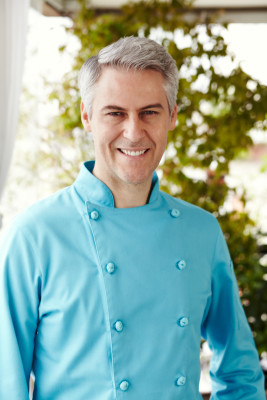
The image is dynamically retrieved the first time this URL is accessed and stored in your Cloudinary account with a public ID of remote_media/commons/2/29/Marcelo_Facini
.
Configuring Auto Upload URL mapping
The Auto Upload feature is configured in the Auto upload mapping section on the Upload tab of the Settings page in Cloudinary's Management Console. In the Auto upload mapping section:
Fill in a Folder name for the Auto upload mapping e.g.
remote_media
.Fill in a URL prefix that will be mapped to the specified folder e.g.
http://upload.wikimedia.org/wikipedia/
.Click the Save button at the bottom of the page.
You can map multiple folder names, each to a different remote URL prefix, by clicking the Add another mapping link. In addition, instead of using a sub-folder, you can map your root folder to a URL prefix by filling in a backslash for the folder name (/
).
As can be seen in the screenshot above, the remote_media
folder is now mapped to the http://upload.wikimedia.org/wikipedia/
URL prefix. Cloudinary delivery URLs including the remote_media
folder prefix can now be accessed, and Cloudinary will automatically retrieve the remote image from the specified Wikimedia URL and store it in your Cloudinary account. From that point on, the image is treated just like any other image that was uploaded to your Cloudinary account.
Auto uploading with on-the-fly image transformations
You can also use the Auto Upload feature to apply any of Cloudinary’s image transformations on-the-fly to the delivered image. Simply add the transformation parameters to the URL directly before the mapped folder name.
For example, the following Cloudinary delivery URL retrieves an image from Wikimedia and stores it in Cloudinary’s demo account, then crops a thumbnail of the original image to a width of 200 pixels and a height of 200 pixels, centers the image on the detected face, makes it circular, applies a sharpen effect - and then delivers that modified image optimized and cached via CDN:
cl_image_tag("remote_media/commons/2/29/Marcelo_Facini.jpg", :width=>200, :height=>200, :gravity=>"face", :radius=>"max", :effect=>"sharpen", :crop=>"thumb")
cl_image_tag("remote_media/commons/2/29/Marcelo_Facini.jpg", array("width"=>200, "height"=>200, "gravity"=>"face", "radius"=>"max", "effect"=>"sharpen", "crop"=>"thumb"))
CloudinaryImage("remote_media/commons/2/29/Marcelo_Facini.jpg").image(width=200, height=200, gravity="face", radius="max", effect="sharpen", crop="thumb")
cloudinary.image("remote_media/commons/2/29/Marcelo_Facini.jpg", {width: 200, height: 200, gravity: "face", radius: "max", effect: "sharpen", crop: "thumb"})
cloudinary.url().transformation(new Transformation().width(200).height(200).gravity("face").radius("max").effect("sharpen").crop("thumb")).imageTag("remote_media/commons/2/29/Marcelo_Facini.jpg")
$.cloudinary.image("remote_media/commons/2/29/Marcelo_Facini.jpg", {width: 200, height: 200, gravity: "face", radius: "max", effect: "sharpen", crop: "thumb"})
cloudinary.Api.UrlImgUp.Transform(new Transformation().Width(200).Height(200).Gravity("face").Radius("max").Effect("sharpen").Crop("thumb")).BuildImageTag("remote_media/commons/2/29/Marcelo_Facini.jpg")

Creating Auto Upload URLs via code
Auto Uploading from within your code can be easily accomplished using Cloudinary's client libraries for all popular development frameworks: Ruby on Rails, PHP, Django, Node.js, Java, .NET, and jQuery. The principle is exactly the same as with any URL/tag building of an uploaded image, except in this case the public ID does not have to match an existing image, but can match a mapped folder name of a remote existing image that will be implicitly fetched on first access. For example, the following code snippet retrieves the same transformed image as shown above:
cl_image_tag("remote_media/commons/2/29/Marcelo_Facini.jpg", :width=>200, :height=>200, :gravity=>"face", :radius=>"max", :effect=>"sharpen", :crop=>"thumb")
cl_image_tag("remote_media/commons/2/29/Marcelo_Facini.jpg", array("width"=>200, "height"=>200, "gravity"=>"face", "radius"=>"max", "effect"=>"sharpen", "crop"=>"thumb"))
CloudinaryImage("remote_media/commons/2/29/Marcelo_Facini.jpg").image(width=200, height=200, gravity="face", radius="max", effect="sharpen", crop="thumb")
cloudinary.image("remote_media/commons/2/29/Marcelo_Facini.jpg", {width: 200, height: 200, gravity: "face", radius: "max", effect: "sharpen", crop: "thumb"})
cloudinary.url().transformation(new Transformation().width(200).height(200).gravity("face").radius("max").effect("sharpen").crop("thumb")).imageTag("remote_media/commons/2/29/Marcelo_Facini.jpg")
$.cloudinary.image("remote_media/commons/2/29/Marcelo_Facini.jpg", {width: 200, height: 200, gravity: "face", radius: "max", effect: "sharpen", crop: "thumb"})
cloudinary.Api.UrlImgUp.Transform(new Transformation().Width(200).Height(200).Gravity("face").Radius("max").Effect("sharpen").Crop("thumb")).BuildImageTag("remote_media/commons/2/29/Marcelo_Facini.jpg")

Auto uploading non-image raw files
While Cloudinary focuses on image management, you can actually automatically upload files of any type to Cloudinary. Cloudinary will store these “raw” files safely in your Cloudinary account, including multiple backups and revision history and deliver these via a CDN. The only change from Auto Uploading images is setting the resource type to raw
instead of image
in the URL as follows:
http://res.cloudinary.com/<your Cloudinary account's cloud name>/raw/upload/<mapped upload folder prefix>/<file>
For example*: To retrieve the following file from Wikimedia, and automatically upload it to your Cloudinary account:
http://upload.wikimedia.org/wikipedia/zh/d/d3/Statistics_for_wikipedia_in_top_ten_languages.xls
Access the following Cloudinary URL:
The file is dynamically retrieved the first time this URL is accessed and stored in your Cloudinary account with an ID of remote_media/zh/d/d3/Statistics_for_wikipedia_in_top_ten_languages.xls
.
To Auto Upload raw files from within your code, set the resource_type parameter to ?raw’. For example, the following code snippet in Node.js builds a URL to fetch the same file as shown above:
cloudinary.url("remote_media/zh/d/d3/Statistics_for_wikipedia_in_top_ten_languages.xls", { resource_type: 'raw' } );
Lazily migrate existing images with Auto Upload
Generally, when migrating to Cloudinary, the simplest method of migration is to upload all your images via Cloudinary's upload API and then update your web application to upload new images to Cloudinary.
The Auto Upload feature offers a powerful alternative method to migrate images within your own existing web application to your Cloudinary account, by dynamically uploading them when first accessed, and without the need to pre-upload them. This “lazy migration” is especially useful if your site has lots of images, some of which may be very rarely accessed - if ever.
Auto Uploaded image URLs share the exact same URL convention as Cloudinary’s regular uploaded image URLs, which means that for each image accessed, Cloudinary first checks whether an image with the given public ID exists in your Cloudinary account, and if it doesn't (and it matches a given Auto Upload folder mapping), it is retrieved on-the-fly from the remote host.
Restricting access to Auto Uploaded images
The Auto Upload examples given so far allow public access to the original image as well as all its derived versions. As an alternative, you can Auto Upload remote images as type private to restrict access to the original image, and/or restrict Auto Uploading images unless they are created explicitly using the authenticated API or signed URLs.
To Auto Upload an image as private, simply set the type as private
instead of image
. This setting prevents access to the original image while allowing access to derived (transformed) versions of the image. For example, the following Java
code snippet uploads new images as private:
Map result = cloudinary.uploader().upload(new File("remote_media/commons/2/29/Marcelo_Facini.jpg"), Cloudinary.asMap("type", "private"));
To restrict images from use with dynamic URLs so that Auto Uploaded images can only be created explicitly using the authenticated API or signed URLs, select the Uploaded option in the Restricted image types section on the Security tab of the Settings page in Cloudinary's Management Console. For example, the following code snippet allows Auto Uploading by signing the URL:
cl_image_tag("remote_media/commons/2/29/Marcelo_Facini.jpg", :sign_url => true)
Auto uploading with Upload presets
When you use Cloudinary's upload API you can leverage many powerful features (eagerly created derived/transformed images, notifications on upload, adding tags and context, requesting moderation and more). To be able to benefit from the same features together with Auto Upload, you can use Cloudinary's Upload Presets for defining the automatic upload actions. Upload Presets include one or more upload parameters, and any of Cloudinary's upload parameters can be defined and included in a preset. Specifying an Upload Preset with the same name as an Auto Upload mapped folder applies all actions and manipulations specified in that Upload Preset to all images uploaded to the mapped Auto Upload folder - even though they are not specified in the simple URL.
For example, naming an Auto Upload mapped folder as remote_media
and also naming an Upload Preset as remote_media
will automatically apply all the actions specified in the Upload Preset when Auto Uploading images to the remote_media
folder.
Auto Upload vs. Fetch
Management - images retrieved with Fetch are managed on your side and just manipulated and delivered by Cloudinary; Auto Uploaded images are managed by Cloudinary like any uploaded image (benefiting from plenty of additional features).
Eager transformations - the option to create multiple variations of the same image during Auto Upload rather than on-the-fly. Also important for manipulating large files and complex transformations (e.g. manipulating animated GIFs).
Upload Presets - automatically apply actions specified in an Upload Preset with the same name as an Auto Upload mapped folder (eagerly creating derived/transformed images, notifications, tagging, moderation and more).
SEO-friendly short URLs - Auto Uploaded URLs do not contain the lengthy URL of the original image.
Access permissions to images - define Auto Uploaded images as private, allow access only to a fixed set of transformations (e.g. keep originals but add a watermark to all viewed versions of it).
Image availability management - with Auto Upload, Cloudinary stores your images (optionally on your own S3), including backups, historical revisions, etc. Fetch URLs are stored on Cloudinary for cache purposes only, which means that you need to continue to support your existing image hosting solution.
Consistency - Auto Uploaded images are treated the same way as any other uploaded image ("newly" uploaded images and "old" images of existing remote URLs are managed identically).