Latest posts
Creating Custom StartPage Extensions
5/5/2010 1:50:00 PM
I've been working on a custom start page sample that creates a new tab on the typical Visual Studio StartPage. This new tab integrates in Bing search functionality, letting developers type in their search query and performing a search. The results can either come up in the Visual Studio internal browser or the user's default browser (depending on a user setting). Along with the search ability, the page also shows the Bing image of the day, so that you can enjoy those scenic images in your favorite IDE. So, if you'd like to see this custom start page in action, you can install it from the VS Gallery.
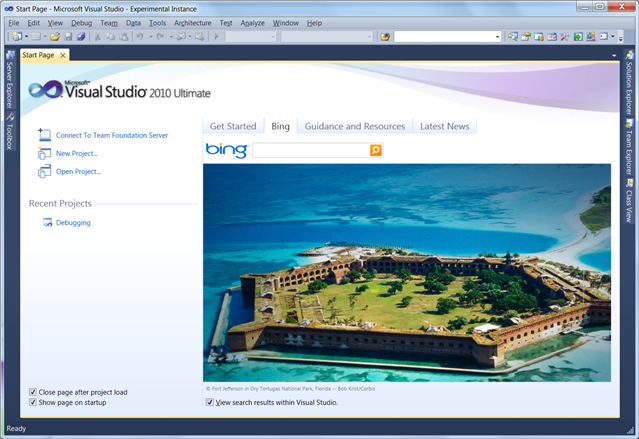
I created this custom StartPage using the Custom Start Page Project Template available on the VS Gallery. This project template lets you quickly set up a start page that is similar in functionality as the Start Page that ships with Visual Studio, along with a new tab for the custom content that you want to add. This allows you to create start pages that fit in well with the VS user interface. But, the start page is all XAML, so you can custom or completely rewrite the start page to look exactly how you wish.
This topic on MSDN gives a good step-by-step process on how to use the Custom Start Page Template.
Along the way while working on this custom start page, I ran across some interesting topics (a couple that I previously blogged about and a good MSDN article):
Overall, using the template makes it really easy to create a full featured start page, and let's you concentrate on implementing the custom functionality you want to surface and not on the infrastructure to get a start page up and running. And, then your just developing a custom WPF control with XAML and code-behind, so it really straightforward. Also, the template automatically builds a .VSIX file for you. This is the new extension deployment framework in Visual Studio 2010, so you can take this .VSIX file and directly upload to the VS Gallery to share you custom start page with the world.
The one drawback of the custom start page deployment is that the new page doesn't automatically get set as your page. You need to go set it yourself. The following steps describe how to switch from the default Start Page to the Bing Start Page.
1.    On the Tools menu, click Options.
2.    On the left side of the Options dialog box, expand the Environment node, and then click Startup.
3.    In the Customize Start Page list, select '[Installed Extension] Bing Start Page'
4.    Click OK.
Â
Cool Extension: Power Commands for VS 2010
5/5/2010 1:21:00 AM
For all of you fans of the Power Commands extension, Lance, from our team, just updated this extension and posted it up on the VS Gallery. This topic contains a full list of all of the new commands that are part of this extension. But, the most interesting ones are:
- Collapse projects
- Open command prompt
- Remove and Sort Usings (recursively)
- Transform templates
Install this extension, and try out some of the other cool commands. You can get this either from the VS Gallery or within Visual Studio 2010 in the Extension Manager (Tools > Extension Manager).
How To: Launch a URL in the Visual Studio Internal Browser
4/26/2010 11:32:00 AM
For the sample extension that I'm working on, I wanted to have the ability to launch a URL in Visual Studio. This is useful for a more integrated experience in the IDE, but of course, some people may prefer to use their default web browser instead. So, I created a method that will either launch the internal or default web browser depending on a user setting (that way users can change to it to match their desired behavior).
For the internal browser, we use the IVsWebBrowsingService.Navigate method. By passing 0 to the dwNavigateFlags, the browsing service reuses an internal browser window if one already exists, or creates a new one if it does not. For the default browser, we call the Process.Start method with the appropriate flags so that it will behave like the managed equivalent of ShellExecute on the URL. Here's the code I wrote for this:
        private const string verbOpen = "Open";
        private IVsWebBrowsingService browserService = null;
       ///Â
        /// Get the IVsWebBrowserService to use for navigating to the internal browser.
        ///Â
       private IVsWebBrowsingService BrowserService
        {
            get
            {
                if (browserService == null)
                {
                    // if we don't already have the internal browser service, get it from the global service provider.
                    browserService = ServiceProvider.GetService(typeof(SVsWebBrowsingService)) as IVsWebBrowsingService;
                }
                return browserService;
            }
        }
        ///Â
        /// Gets the initialized instance of ProcessStartInfo for a ShellExecute Open command.
        ///Â
        public ProcessStartInfo StartInfo
        {
            get
            {
                if (startInfo == null)
                {
                    startInfo = new ProcessStartInfo();
                    startInfo.UseShellExecute = true;
                    startInfo.Verb = verbOpen;
                }
Â
                return startInfo;
            }
        }
        ///Â
        /// Launches the specified Url either in the internal VS browser or the
        /// user's default web browser.
        ///Â
        /// VS's browser service for interacting with the internal browser.
        /// Url to launch.
        /// true to use the internal browser; false to use the default browser.
        private void LaunchWebBrowser(IVsWebBrowsingService browserService, string launchUrl, bool useInternalBrowser)
        {
            try
            {
                if (useInternalBrowser == true)
                {
                    // if set to use internal browser, then navigate via the browser service.
                    IVsWindowFrame ppFrame;
Â
                    // passing 0 to the NavigateFlags allows the browser service to reuse open instances
                    // of the internal browser.
                    browserService.Navigate(launchUrl, 0, out ppFrame);
                }
                else
                {
                    // if not, launch the user's default browser by starting a new one.
                    StartInfo.FileName = launchUrl;
                    Process.Start(StartInfo);
                }
            }
            catch
            {
                // if the process could not be started, show an error.
                MessageBox.Show("Cannot launch this url.", "Extension Error", MessageBoxButton.OK, MessageBoxImage.Error);
            }
        }
Â
 The code above gets the IVsWebBrowsingService by querying a service provider. You can get a service provider in your extension by various methods: for typical extension look in this MSDN article; or if doing it from a StartPage, read my previous article on that subject.
Note: If you look at the IVsWebBrowsingService interface, you will notice it defines a CreateExternalWebBrowser method. I first thought I would use this method, since it was defined on this service. However, when I tried to call it, this method returned E_NOTIMPL. It appears this implementation has been removed, but calling ShellExecute on a URL is easy enough to do.
Â
How To: Get a ServiceProvider from the VS Start Page
4/21/2010 5:16:00 PM
When working with Visual Studio extensibility, getting a ServiceProvider is the main way to find and use services that are available in Visual Studio. The new VS Start Page is written in WPF and getting to a ServiceProvider from within the page or one of its child controls is not easily found. There is a fairly good topic on this in MSDN: http://msdn.microsoft.com/en-us/library/ff432842(v=VS.100).aspx, though the nugget about getting to the ServiceProvider is a little lost in the full article. So, I decided to pull it out into a post here to make it easier to find this useful snippet.
First, use the Custom StartPage template to create a new project to work with (this topic explains how to use that template).
Then, in the code file for your UserControl, you can add the following code as a property of the control class:
       private DTE2 dte = null;
       private IServiceProvider serviceProvider = null;
Â
       ///
       /// Gets the StartPage's DTE object from its DataContext.
       ///
       private DTE2 DTE
       {
           get
           {
               if (dte == null)
               {
                   // if we don't already have the ServiceProvider, get it from the page's DataContext.
                   ICustomTypeDescriptor typeDescriptor = DataContext as ICustomTypeDescriptor;
                   PropertyDescriptorCollection propertyCollection = typeDescriptor.GetProperties();
Â
                   // first get the DTE object from the DataContext.
                   dte = propertyCollection.Find("DTE", false).GetValue(DataContext) as DTE2;
               }
Â
               return dte;
           }
      }
Â
       ///
       /// Gets the StartPage's ServiceProvider for use in our custom control.
       ///
       private IServiceProvider ServiceProvider
       {
           get
           {
               if (serviceProvider == null)
               {
                   // get and then cast the DTE object to a ServiceProvider.
                   serviceProvider = new ServiceProvider((Microsoft.VisualStudio.OLE.Interop.IServiceProvider)DTE);
               }
Â
               return serviceProvider;
           }
       }
Note: this code requires you to include the following namespaces in your source file: EnvDTE80, Microsoft.VisualStudio.Shell, Microsoft.VisualStudio.Shell.Interop, and System.ComponentModel.
Now that you have this method to get at the ServiceProvider from your custom StartPage, you can use it to get any of the services provided by Visual Studio and integrate them into your page.
Â
Cool Extension: Custom Start Page Template
4/19/2010 4:51:00 PM
For those that have seen Visual Studio 2010, there is a fancy new start page that is much more functional and integrated with the product. Part of this new Start Page infrastructure is the ability to replace the one from the product with a custom page. And, this extension gives you the tools to start creating and sharing your own custom Start Pages.Â
I was just trying out the Custom Start Page template that Greg from our team posted up on the Visual Studio Gallery. So far, so good... I was able to create a new start page that looks similar to the one in Visual Studio but added a small bit of functionality (an additional tab). The template is fairly self-explanatory and has a good F5 experience for building and debugging the custom Start Page in an experimental instance of VS. Also, the project builds into a VSIX file that can be uploaded to the VS Gallery to easily share with others.
I'll post my start page changes when I get it wrapped up a little
Â